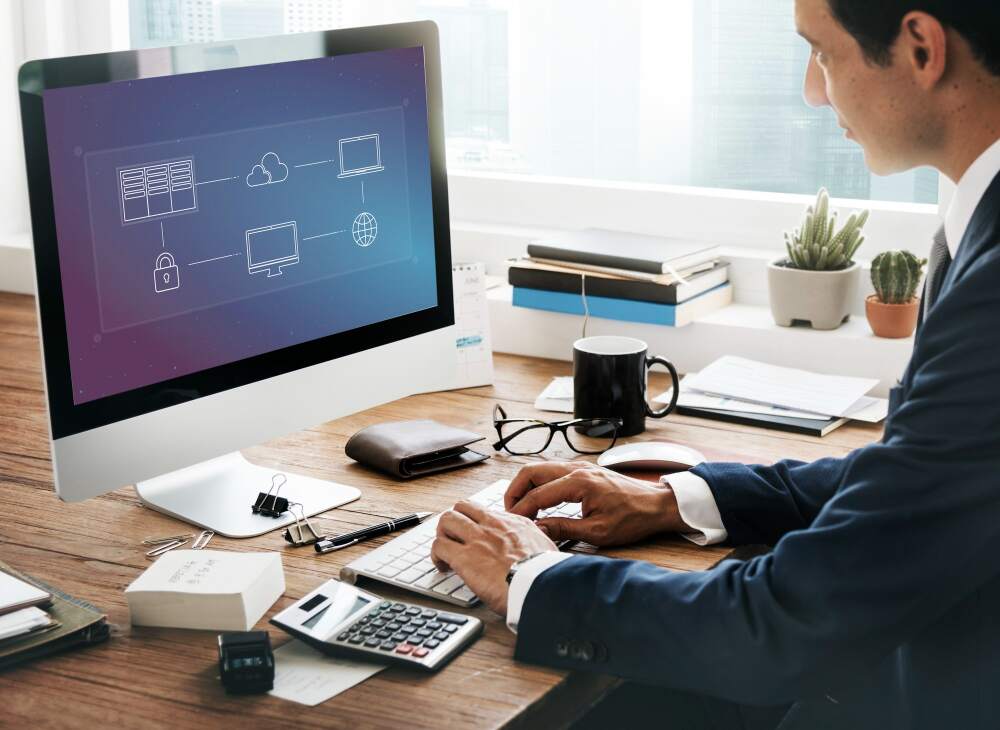
Definition of File Input and Output
File Input and Output (I/O) in C is a way for a program to interact with files on your computer. Think of it like reading and writing data to and from a text file.
1. Reading from a File (File Input):
To read data from a file in C, you need to follow these basic steps:
#include <stdio.h>
int main() {
FILE *filePointer;
char data[100]; //Assuming a maximum of 100 character in a line
//Open the file for reading
filePointer = fopen(“example.txt” , “r”);
//Check if the file opened successfully
if (filePointer == NULL) {
printf(“File not found or unable to open. \n”);
return 1: //Exit the program with an error code
}
//Read and print data from the file
while (fgets(data, sizeof(data), filePointer) != NULL) {
printf(“%s” , data);
}
// Close the file
fclose(filePointer);
return 0;
}
This program opens a file named “example.txt” for reading (“r” mode), reads its content line by line, and prints it on the console.
2. Writing to a File (File Output):
To write data to a file in C, you can use the following example:
#include <stdio.h>
int main() {
FILE *filePointer;
// Open the file for writing (creates a new file or overwrites an existing one)
filePointer = fopen(“output.txt”, “w”);
// Check if the file opened successfully
if (filePointer == NULL) {
printf(“Unable to create or open the file.\n”);
return 1;
}
// Write data to the file
fprintf(filePointer, “Hello, this is written to the file!\n”);
// Close the file
fclose(filePointer);
return 0;
}
This program creates a new file named “output.txt” for writing (“w” mode) and writes a line to it.
Overview of File Handling in C
File handling in C allows you to perform operations on files, such as reading from them or writing to them. This is essential for storing and retrieving data persistently. In C, file handling is done through a set of functions provided by the standard I/O library.
Here are the basic steps involved in file handling:
- Include the Necessary Header File:
- To use file handling functions in C, you need to include the <stdio.h> header file, which stands for standard input/output.
- #include <stdio.h>
- File Pointers:
In C, a file is represented by a file pointer. A file pointer is a special variable that keeps track of the file being accessed. You need to declare a file pointer before using it.
FILE *filePointer;
- Opening a File:
To perform operations on a file, you need to open it first. The fopen() function is used for this purpose. It returns a file pointer that you will use for subsequent operations.
filePointer = fopen(“example.txt”, “r”); // Opens “example.txt” for reading
The second argument specifies the mode: “r” for reading, “w” for writing, “a” for appending, and so on.
- Reading from a File:
The fscanf() function is used to read data from a file, similar to scanf() for input from the keyboard.
int data;
fscanf(filePointer, “%d”, &data); // Reads an integer from the file
- Writing to a File:
To write data to a file, you use the fprintf() function, which is similar to printf().
fprintf(filePointer, “Hello, File Handling!”);
- Closing a File:
After performing operations on a file, it’s important to close it using the fclose() function.
fclose(filePointer);
- This step is crucial as it ensures that any changes made to the file are saved, and system resources are released.
Example:
Let’s consider a simple example where we read and write to a file:
#include <stdio.h>
int main() {
FILE *filePointer;
int number;
// Opening a file for writing
filePointer = fopen(“data.txt”, “w”);
// Writing to the file
fprintf(filePointer, “42”);
// Closing the file
fclose(filePointer);
// Opening the file for reading
filePointer = fopen(“data.txt”, “r”);
// Reading from the file
fscanf(filePointer, “%d”, &number);
// Displaying the read data
printf(“Number from file: %d\n”, number);
// Closing the file
fclose(filePointer);
return 0;
}
This program writes the number 42 to a file and then reads it back, demonstrating the basics of file handling in C.
Error Handling in File Input
When you are reading data from a file, you need to make sure that the file exists and can be opened successfully. Here’s a basic example using fopen and checking for errors:
#include <stdio.h>
int main() {
FILE *filePointer;
char fileName[] = “input.txt”;
// Open the file for reading
filePointer = fopen(fileName, “r”);
// Check if the file was opened successfully
if (filePointer == NULL) {
printf(“Error opening file for reading.\n”);
return 1; // Return an error code
}
// Read data from the file
// Close the file when done
fclose(filePointer);
return 0;
}
In this example, if the file “input.txt” doesn’t exist or if there’s any issue opening the file, an error message is displayed.
Error Handling in File Output:
Similarly, when writing data to a file, you need to check if the file can be opened for writing. Here’s an example:
#include <stdio.h>
int main() {
FILE *filePointer;
char fileName[] = “output.txt”;
// Open the file for writing
filePointer = fopen(fileName, “w”);
// Check if the file was opened successfully
if (filePointer == NULL) {
printf(“Error opening file for writing.\n”);
return 1; // Return an error code
}
// Write data to the file
// Close the file when done
fclose(filePointer);
return 0;
}
In this example, if the file “output.txt” can’t be opened for writing, an error message is displayed.
These checks are important because they help prevent your program from crashing or behaving unexpectedly when it encounters file-related issues. Always remember to close the file using fclose when you are done working with it.