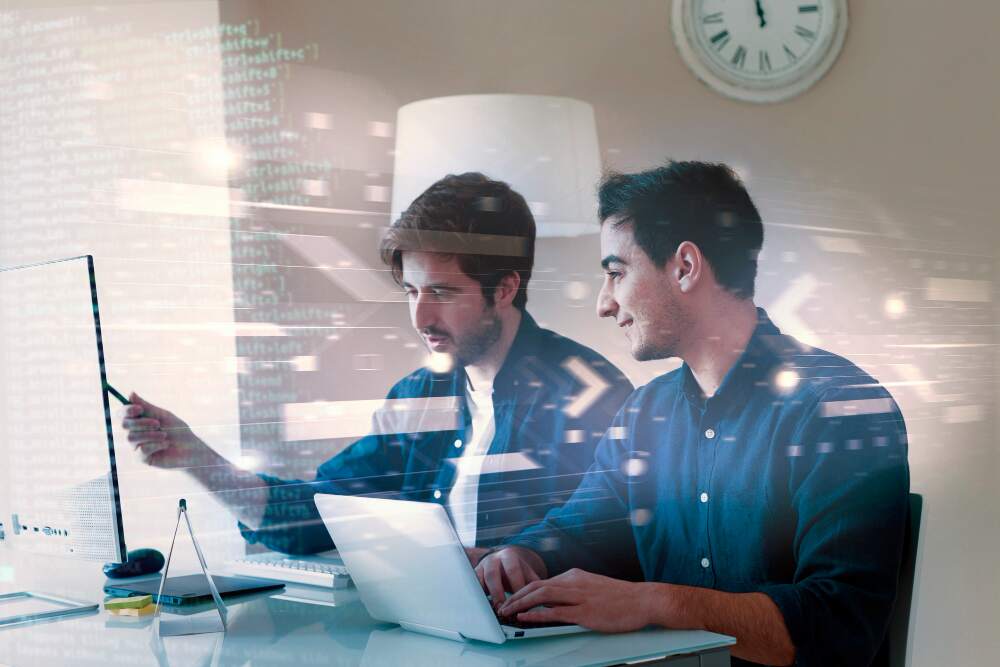
Linked lists are a fundamental data structure in computer science, and mastering them is crucial for any aspiring programmer. In this comprehensive guide, we’ll explore the world of linked lists using the Python 3 programming language. Get ready to unlock the power of data organization through clear explanations and practical examples.
Understanding Linked Lists:
Imagine you have a chain of linked items, much like a train where each carriage is connected to the next. In a linked list, we have nodes, and each node contains two parts: data and a reference (or link) to the next node in the sequence.
Let’s create a simple linked list in Python:
class Node:
def __init__(self, data=None):
self.data = data
self.next_node = None
# Creating nodes
node1 = Node(“apple”)
node2 = Node(“banana”)
node3 = Node(“cherry”)
# Linking nodes
node1.next_node = node2
node2.next_node = node3
Types of Linked Lists
There are several variations of linked lists that serve different purposes based on the specific requirements of a program. The most common types of linked lists are:
- Singly Linked List: In a singly linked list, each node has a reference to the next node, forming a unidirectional chain. This is the simplest form of a linked list and is commonly used in many applications.
- Doubly Linked List: In a doubly linked list, each node has references to both the next and previous nodes, creating a bidirectional chain. This allows for easier traversal in both directions but requires more memory to store the additional references.
- Circular Linked List: In a circular linked list, the last node of the list contains a reference to the first node, creating a circular structure. This can be useful in scenarios where continuous looping through the elements is required.
Implementing the Linked List Structure
To implement a linked list in Python 3, we can define a class for the nodes and another class for the linked list itself. Let’s take a look at a basic implementation:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
In this implementation, the Node class represents each element in the linked list. It has a data attribute to store the value of the node and a next attribute to reference the next node in the list. The LinkedList class serves as a wrapper for the nodes and contains a head attribute, which points to the first node in the list.
Adding Elements to a Linked List
Adding elements to a linked list involves creating a new node and updating the appropriate references. Let’s consider two scenarios: adding an element at the beginning of the list and adding an element at the end of the list.
Adding an Element at the Beginning
To add an element at the beginning of a linked list, we need to create a new node, assign its next reference to the current head of the list, and update the head to point to the new node. Here’s an example implementation:
def add_at_beginning(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
Adding an Element at the End
To add an element at the end of a linked list, we need to traverse the list until we reach the last node, create a new node, and update the next reference of the last node to point to the new node. Here’s an example implementation:
def add_at_end(self, data):
new_node = Node(data)
if self.head is None: # If the list is empty
self.head = new_node
else:
current = self.head
while current.next is not None:
current = current.next
current.next = new_node
Traversing a Linked List
Traversing a linked list involves visiting each node in the list and accessing its data. This can be done by starting from the head node and following the next references until we reach the end of the list. Here’s an example implementation:
def traverse(self):
current = self.head
while current is not None:
print(current.data)
current = current.next
Updating Elements in a Linked List
Updating elements in a linked list requires finding the specific node that needs to be updated and modifying its data. This can be done by traversing the list, comparing the data of each node with the target value, and updating it when a match is found. Here’s an example implementation that updates the first occurrence of a target value:
def update(self, target, new_data):
current = self.head
while current is not None:
if current.data == target:
current.data = new_data
break
current = current.next
By following these implementations, you can effectively create and manipulate linked lists in Python 3. Whether you need to efficiently store and organize large amounts of data or implement complex data structures, linked lists provide a powerful tool to manage information dynamically. Start leveraging the power of data organization unleashed by implementing linked lists in Python 3 today!
“Linked lists offer a refreshing twist in the world of data organization. With their dynamic nature and flexibility, they can bring a whole new level of efficiency to your programs.”
Conclusion:
Linked lists are powerful tools for organizing data dynamically. By understanding the basics and practising with Python, you’ll be well-equipped to tackle more complex data structures.