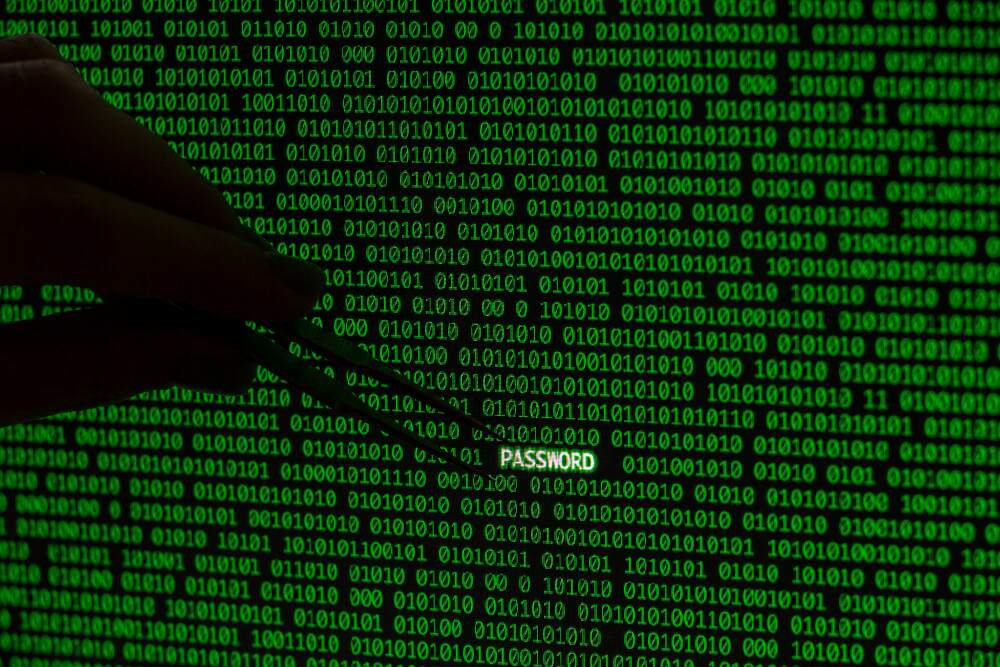
Understanding Binary Search
Binary Search is like a smart way of finding a particular item in a sorted list. Imagine you have a phone book with names in alphabetical order, and you want to find a specific person. Instead of going page by page, you start in the middle. If the name you’re looking for comes before the middle, you know it must be in the first half. If it comes after, it’s in the second half. You keep doing this, narrowing down your search until you find the name.
In Java, we can implement Binary Search in an array, which is like a list. Here’s a simple example:
public class BinarySearchExample {
// Binary Search method
static int binarySearch(int arr[], int target) {
int left = 0, right = arr.length – 1;
while (left <= right) {
int mid = left + (right – left) / 2;
// Check if target is present at mid
if (arr[mid] == target)
return mid;
// If target is greater, ignore the left half
if (arr[mid] < target)
left = mid + 1;
// If target is smaller, ignore the right half
else
right = mid – 1;
}
// Target is not present in array
return -1;
}
public static void main(String[] args) {
int[] sortedArray = { 2, 5, 8, 12, 16, 23, 38, 45, 56, 72 };
int targetElement = 23;
int result = binarySearch(sortedArray, targetElement);
if (result == -1)
System.out.println(“Element not present in the array”);
else
System.out.println(“Element found at index ” + result);
}
}
In this Java program:
- binarySearch is the method where the magic happens.
- We maintain two pointers, left and right, which represent the current search space.
- We calculate the mid index and compare the element at that index with the target.
- Depending on the result, we update left or right, narrowing down the search space.
- We repeat this process until we find the target or determine it’s not in the array.
This is how Binary Search works in a nutshell! It’s an efficient way to find an element in a sorted list without checking each element one by one.
Basic Concepts of Binary Search
Binary search operates on the principle of divide and conquer. Given a sorted array, it starts by examining the middle element. If the desired element is found, the search terminates. Otherwise, if the middle element is greater than the desired element, it continues the search on the left half of the array. Conversely, if the middle element is smaller, the search proceeds on the right half. This process is repeated until the element is found or the search space is reduced to zero.
Implementing Binary Search in Java
To implement binary search in Java, we can use a recursive or iterative approach. The recursive approach involves defining a helper method that takes the array, target element, start index, and end index as parameters. The base case checks if the start index is greater than the end index, indicating that the element is not present. Otherwise, it calculates the middle index and compares the middle element with the target element. Based on the result, it recursively calls itself on the appropriate half of the array.
On the other hand, the iterative approach uses a while loop to iterate until the start index becomes greater than the end index. Within the loop, it calculates the middle index and compares it with the target element. Based on the result, it updates the start or end index accordingly, effectively reducing the search space. The loop terminates when the element is found or the search space is empty.
Step-by-Step Guide to Binary Search Algorithm
Let’s delve into a step-by-step guide on how the binary search algorithm works in Java:
- Start with a sorted array and define the target element.
- Set the start index as 0 and the end index as the length of the array minus one.
- Calculate the middle index using the formula: (start + end) / 2.
- Compare the middle element with the target element.
- If they are equal, the element is found. Return the index.
- If the middle element is greater, update the end index to (middle – 1).
- If the middle element is smaller, update the start index to (middle + 1).
- Repeat steps 3 to 7 until the element is found or the search space is empty.
Optimizing Binary Search for Efficiency
While binary search is already an efficient algorithm, there are several techniques we can apply to further optimize its performance.
1. Sorting the Array
Binary search requires a sorted array as input. Therefore, it is crucial to ensure that the array is sorted before applying the binary search algorithm. Sorting the array beforehand eliminates the need for additional checks and ensures the search space is properly divided.
2. Midpoint Calculation
In some cases, calculating the midpoint using (start + end) / 2 may result in an overflow when dealing with large arrays. To prevent this, we can use the formula start + (end – start) / 2 to calculate the midpoint. This formula guarantees accurate results while avoiding potential overflow issues.
3. Avoiding Redundant Comparisons
During each iteration, binary search compares the middle element with the target element. To optimize the algorithm, we can modify the comparison step to avoid redundant comparisons. By comparing the middle element only once and storing it in a temporary variable, we can use it for subsequent comparisons. This optimization reduces unnecessary calculations and improves overall performance.
Dealing with Duplicates in Binary Search
Binary search assumes that the array does not contain any duplicate elements. However, what if duplicates are present? There are two common approaches to handling duplicates in binary search:
1. Finding the First Occurrence
If the goal is to find the first occurrence of the target element, we can modify the binary search algorithm slightly. When the middle element is equal to the target element, we continue the search on the left half of the array instead of terminating. This allows us to find the first occurrence of the element.
2. Finding the Last Occurrence
Similarly, if we need to find the last occurrence of the target element, we can modify the algorithm accordingly. When the middle element is equal to the target element, we continue the search on the right half of the array. By doing so, we can identify the last occurrence of the element.
Variations of Binary Search
Binary search is a versatile algorithm that can be adapted to solve various problems beyond simple element search. Here are some notable variations:
1. Binary Search on Rotated Arrays
In certain scenarios, the array might be rotated or shifted, making it no longer strictly sorted. To handle this, we can apply a modified binary search algorithm that accounts for the rotation. By adjusting the start and end indices based on specific conditions, we can still efficiently find the target element.
2. Binary Search Trees
Binary search trees (BSTs) are data structures that leverage the principles of binary search. Each node in a BST has a value and two child nodes – a left child with smaller values and a right child with larger values. BSTs provide efficient insertion, deletion, and search operations. By maintaining the binary search property, BSTs enable quick retrieval of elements.
Binary Search vs Linear Search: A Comparison
Now that we have explored binary search in depth, let’s compare it with linear search to understand the advantages and disadvantages of each:
Binary search excels when dealing with large sorted arrays. By dividing the search space in half with each iteration, it quickly narrows down the possibilities and achieves logarithmic time complexity of O(log n). On the other hand, linear search sequentially compares each element with the target element and has a linear time complexity of O(n).
While binary search offers faster retrieval, it requires a sorted array as input. In contrast, linear search works on unsorted arrays and does not have any preconditions. Additionally, binary search is not suitable for dynamic collections where insertions and deletions frequently occur, as maintaining the sorted order becomes costly.
In summary, binary search is ideal for sorted arrays with infrequent modifications, providing significant speed improvements over linear search. However, for small, unsorted arrays or cases where the data is constantly changing, linear search may be a more practical choice.
With this expert guide, you have gained a comprehensive understanding of binary search in Java. Armed with this knowledge, you can confidently apply binary search to solve a variety of search problems efficiently.