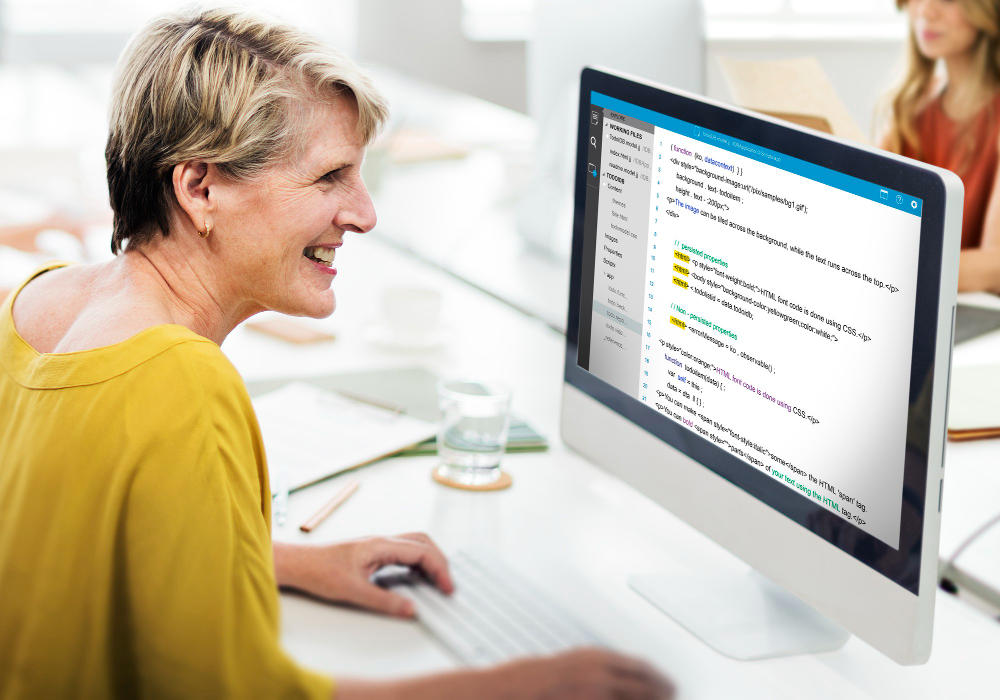
In this article, we delve into the intricate world of handling user input in Python. As any programmer knows, efficient input handling is crucial for seamless program execution. We will explore the concept of input lists and how to wield them effectively in your Python code. From understanding the basics to conquering complex input scenarios, this guide will equip you with the knowledge to streamline your user input processes. Get ready to revolutionize your Python programming skills and witness enhanced input handling prowess. Prepare to conquer the world of user input like never before!
What’s a List?:
A list in Python is like a container that holds multiple pieces of information. Imagine it as a shopping list where you write down different items you want to buy. In programming, we use lists to store and organize data.
Why Input Lists?:
Now, let’s say we want the user to give us several pieces of information at once. Instead of asking one question at a time, like “What’s your name?” and then “What’s your age?”, we can use a list to gather all the answers together.
How to Use Input Lists in Python:
- Getting Ready:
- We start by creating an empty list. It’s like having an empty basket before you start shopping.
- user_responses = []
- Asking Questions:
- We use a loop to ask the user questions. Each answer goes into our list.
- for i in range(3): # Let’s ask 3 questions
- answer = input(“Enter your answer: “)
- user_responses.append(answer)
- Results:
- Now, our user_responses list has all the answers neatly stored.
- print(“User Responses:”, user_responses)
Example:
Let’s say we ask three questions – name, age, and favorite color. The user types in their answers, and our list looks like this:
User Responses: [‘John’, ’22’, ‘Blue’]
Understanding Input Lists in Python
Input lists are a fundamental concept in Python programming that allow efficient handling of user input. A list is a versatile data structure that can hold multiple values, making it suitable for storing and managing user inputs. In Python, lists are denoted by square brackets and can contain elements of different data types.One creative aspect of using input lists is the ability to gather diverse information from users in a structured manner. By utilizing lists, you can prompt users for various inputs such as names, ages, or even complex data like coordinates or preferences. This flexibility empowers developers to design interactive programs that cater to different user requirements.
To create a list, you simply put your items inside square brackets [] and separate them with commas. For example:
my_list = [1, 2, 3, 4, 5]
Taking Input into a List
Now, let’s make it more interesting. Instead of hardcoding values into a list, we can take input from the user. Python provides a built-in function called input() that allows us to get input from the user.
Here’s a simple program to take three numbers as input and store them in a list:
# Taking input for a list
num1 = int(input(“Enter the first number: “))
num2 = int(input(“Enter the second number: “))
num3 = int(input(“Enter the third number: “))
# Creating a list with the input values
my_list = [num1, num2, num3]
# Displaying the list
print(“Your list is:”, my_list)
In this example:
- input() is used to get input from the user.
- int() is used to convert the input (which is initially a string) into an integer.
- We create a list named my_list containing the three input numbers.
- Finally, we print out the resulting list.
Benefits of Efficient User Input Handling
- 1.Accuracy:
Efficient input handling ensures that the program accurately captures what the user is trying to communicate. It’s like having a conversation where both sides understand each other well.
- 2.Preventing Errors:
When users type something unexpected, like letters instead of numbers, a well-handled input will catch these mistakes and guide the user to input the correct information. It’s like a helpful friend who gently corrects you when you make a small mistake.
- 3.User-Friendly Experience:
Good input handling makes your program more user-friendly. Users appreciate when a program gives clear instructions and understands their inputs easily, making the whole experience smoother and enjoyable.
- 4.Efficient Execution:
Imagine your program as a chef in a kitchen. Efficient input handling ensures that the chef (your program) gets the right ingredients (user inputs) in the right format, allowing it to cook (execute) faster and without any confusion.
- 5.Customization:
Well-handled user input allows users to customize their interactions. For example, if you’re writing a game, users might want to choose their character’s name or color. Efficient input handling makes it easy for users to personalize their experience.
- 6.Debugging and Maintenance:
When your program understands user input well, it becomes easier to find and fix issues. It’s like having a well-organized book – if there’s an error, you can quickly identify and correct it without flipping through pages.
Reading User Input and Appending to the List
In programming, we often need to take input from the user and store it for further use. Think of it like asking a question and getting an answer from someone. In Python, we use a combination of functions to do this.
Reading User Input:
In Python, the input() function is like a microphone that allows the program to listen to what the user types. Here’s a simple example:
# Asking the user for their name
user_name = input(“Enter your name: “)
# Printing a greeting with the user’s name
print(“Hello, ” + user_name + “!”)
In this example, the input(“Enter your name: “) part prompts the user to type their name, and whatever they type is stored in the variable user_name. We then print a greeting using their name.
Appending to the List:
Now, let’s talk about lists. A list in Python is like a container where you can put multiple pieces of information. It’s like having a shopping list where you add items one by one.
# Creating an empty list
my_list = []
# Adding items to the list using append()
my_list.append(“Apple”)
my_list.append(“Banana”)
my_list.append(“Orange”)
# Printing the updated list
print(“My fruit list:”, my_list)
Putting It Together:
Now, let’s combine reading user input and appending to a list. Imagine we want to create a list of names entered by the user:
# Creating an empty list
name_list = []
# Reading user input and appending to the list
name1 = input(“Enter the first name: “)
name_list.append(name1)
name2 = input(“Enter the second name: “)
name_list.append(name2)
# Printing the final list of names
print(“List of names:”, name_list)
Here, we use the input() function to get names from the user and then use append() to add each name to the name_list.
Ensuring Valid Input using Error Handling
Handling user input is a critical aspect of developing robust Python programs. To ensure that the input lists are filled with valid and expected values, error handling techniques come into play. By proactively anticipating and managing potential errors, we can create a smoother user experience and prevent program crashes or unintended consequences.
In Python, we can use something called “try-except” blocks to handle errors gracefully. Here’s a simple example:
try:
# Get the number of eggs from the user
eggs = int(input(“Enter the number of eggs: “))
# If the input is not a number, this line won’t be executed
# We’ll handle the error if the input is not a number in the except block
print(“You entered:”, eggs)
except ValueError:
# This block will run if there’s an error, specifically if the input is not a number
print(“Oops! That’s not a valid number. Please enter a valid number.”)
Let’s break this down:
- We use try: to enclose the code where potential errors might occur.
- The user is prompted to enter the number of eggs.
- int(input(…)) tries to convert the input to an integer. If the input is not a valid number, a ValueError occurs.
- If there’s a ValueError, the program jumps to the except ValueError: block.
- Inside the except block, we print an error message and prompt the user to enter a valid number.
Looping through the Input List
Let’s say you have a list of your favorite fruits: fruits = [‘apple’, ‘banana’, ‘orange’, ‘grape’]. Now, you want to print each fruit one by one.
Using a Loop in Python:
In Python, we often use a for loop for this kind of task. Here’s how you can loop through the list of fruits:
# Define the list of fruits
fruits = [‘apple’, ‘banana’, ‘orange’, ‘grape’]
# Loop through each fruit in the list
for fruit in fruits:
print(fruit)
Explanation:
- for fruit in fruits: – This line sets up the loop. It says, “for each item in the ‘fruits’ list, do the following:”
- print(fruit) – This line is indented, indicating that it’s part of the loop. It prints the current fruit in the list during each iteration of the loop.
Output:
apple
banana
orange
grape
Breaking Down the Loop:
- The loop starts with the first fruit in the list (‘apple’), prints it, then moves to the next fruit (‘banana’), and so on, until all fruits in the list are printed.
Key Takeaways:
- A loop helps you avoid repeating the same code for each item in a list.
- The for item in list: syntax is commonly used to iterate through elements in a list.
- The indented block of code below the loop definition is what gets executed during each iteration.
Conclusion
In conclusion, mastering the art of efficiently handling user input in Python through input lists opens up a world of possibilities for developers. By following the best practices discussed in this article, you can enhance the user experience by ensuring accurate and convenient data entry. Moreover, utilizing error handling techniques allows for graceful recovery from erroneous inputs, promoting robustness in your programs. So, embrace the power of input lists and let your code interface seamlessly with users, creating a harmonious and satisfying interactive environment.