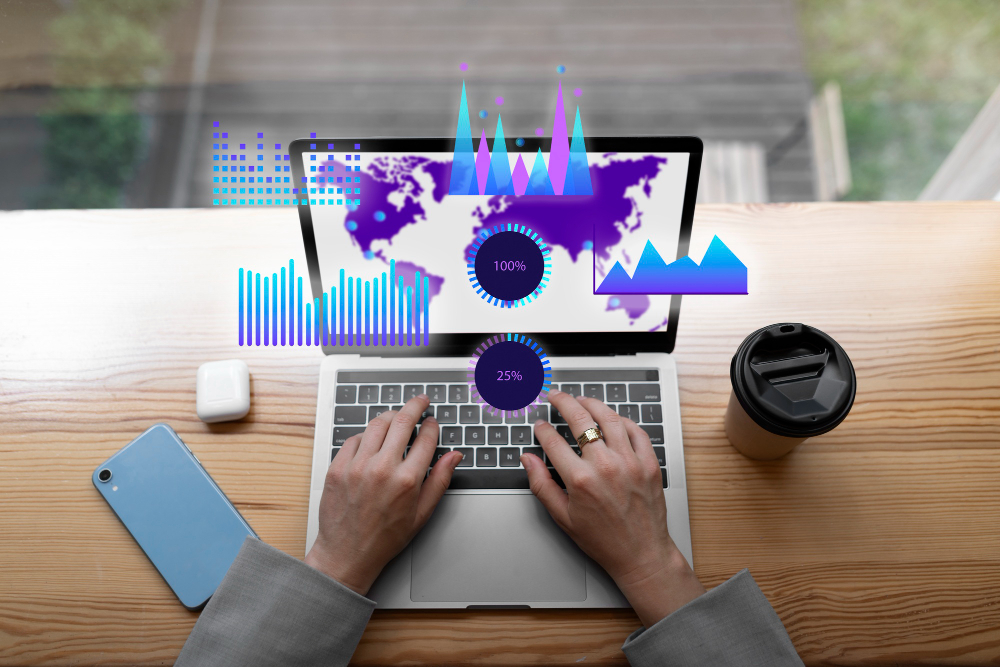
Bubble sort is a simple sorting algorithm that repeatedly steps through a list, compares adjacent elements, and swaps them if they are in the wrong order. It is a basic sorting technique that is useful in understanding data structure and algorithms. Bubble sort is one of the most common algorithms used in the “Data Structures and Algorithms with C++” course. In this article, we will discuss how bubble sort works, its time complexity, and its space complexity. We will also include C++ code to demonstrate each step of the sorting process.
Understanding Bubble Sort
Bubble Sort is a way of sorting elements in a list or an array. The idea is to compare pairs of adjacent elements and swap them if they are in the wrong order. This process is repeated until the entire list is sorted.
Example in C++:
Let’s take a simple example to sort an array of numbers using Bubble Sort in C++.
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// Swap the elements if they are in the wrong order
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
int main() {
int arr[] = {64, 25, 12, 22, 11};
int n = sizeof(arr)/sizeof(arr[0]);
cout << “Original array: “;
for (int i = 0; i < n; i++) {
cout << arr[i] << ” “;
}
bubbleSort(arr, n);
cout << “\nSorted array: “;
for (int i = 0; i < n; i++) {
cout << arr[i] << ” “;
}
return 0;
}
Analyzing the Performance of Bubble Sorting
Imagine you have a list of numbers, and you want to arrange them in ascending order (from the smallest to the largest). Bubble Sort is a simple sorting algorithm that can help you achieve this. Let’s go through the steps with an example in C++.
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n – 1; i++) {
for (int j = 0; j < n – i – 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap if the current element is greater than the next
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
int numbers[] = {5, 2, 9, 1, 5};
int size = sizeof(numbers) / sizeof(numbers[0]);
cout << “Original Array: “;
for (int i = 0; i < size; i++) {
cout << numbers[i] << ” “;
}
// Call the bubbleSort function to sort the array
bubbleSort(numbers, size);
cout << “\nSorted Array: “;
for (int i = 0; i < size; i++) {
cout << numbers[i] << ” “;
}
return 0;
}
Understanding the Performance:
Bubble Sort works by repeatedly swapping adjacent elements if they are in the wrong order. Here’s a simple breakdown:
- Outer Loop (i):
- It goes through the entire array.
- In each pass, the largest unsorted element “bubbles up” to its correct position.
- Inner Loop (j):
- Compares adjacent elements and swaps them if they are in the wrong order.
- Performance Analysis:
- Bubble Sort has a time complexity of O(n^2), meaning it may not be efficient for large datasets.
- It’s good for educational purposes but not the best choice for real-world scenarios.
Applying Optimization Techniques to Bubble Sorting
Bubble sort is like organizing a line of students from shortest to tallest. You start at one end of the line and compare the height of the first student with the one next to them. If the first student is shorter, you swap their positions. Then you move to the next pair of students and do the same thing. You keep doing this until you reach the end of the line. This process is like one pass of bubble sort.
Optimization Techniques:
Now, let’s talk about making this process a bit smarter or faster.
- Early Stop:
- Imagine if, after a pass through the line, nobody changed places. This means everyone is already in the right order, so you can stop sorting. This is like realizing you don’t need to keep checking if the line is already sorted.
- Optimized Swapping:
- Instead of swapping students immediately when you find a pair out of order, you can just remember that there was an out-of-order pair. Once you finish a pass through the line, you go back and swap only the pairs that were out of order. This can save some unnecessary swapping.
Example in C++:
Let’s look at a simple C++ code snippet for optimized bubble sort:
#include <iostream>
using namespace std;
void optimizedBubbleSort(int arr[], int n) {
bool swapped;
for (int i = 0; i < n – 1; i++) {
swapped = false;
for (int j = 0; j < n – i – 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap the elements if they are in the wrong order
swap(arr[j], arr[j + 1]);
swapped = true;
}
}
// If no two elements were swapped in inner loop, the array is sorted
if (!swapped)
break;
}
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr) / sizeof(arr[0]);
optimizedBubbleSort(arr, n);
cout << “Sorted array: “;
for (int i = 0; i < n; i++)
cout << arr[i] << ” “;
return 0;
}
This C++ code includes an optimized version of the bubble sort algorithm. It incorporates the early stop and optimized swapping techniques we discussed.
Conclusion
In conclusion, the art of maximizing performance with bubble sorting unravels a multitude of tips and tricks that can truly revolutionize your data processing endeavors. By diligently applying the discussed optimization techniques, such as enhancing efficiency, managing memory usage, and optimizing for large datasets, you will unlock the true potential of bubble sorting. Moreover, by exploring alternative sorting algorithms and leveraging external libraries to streamline your data processing tasks, you will further expand your horizons in the realm of data manipulation. Embrace these strategies with an unwavering spirit and witness how even the simplest sorting technique can accelerate your journey towards faster and more efficient data processing