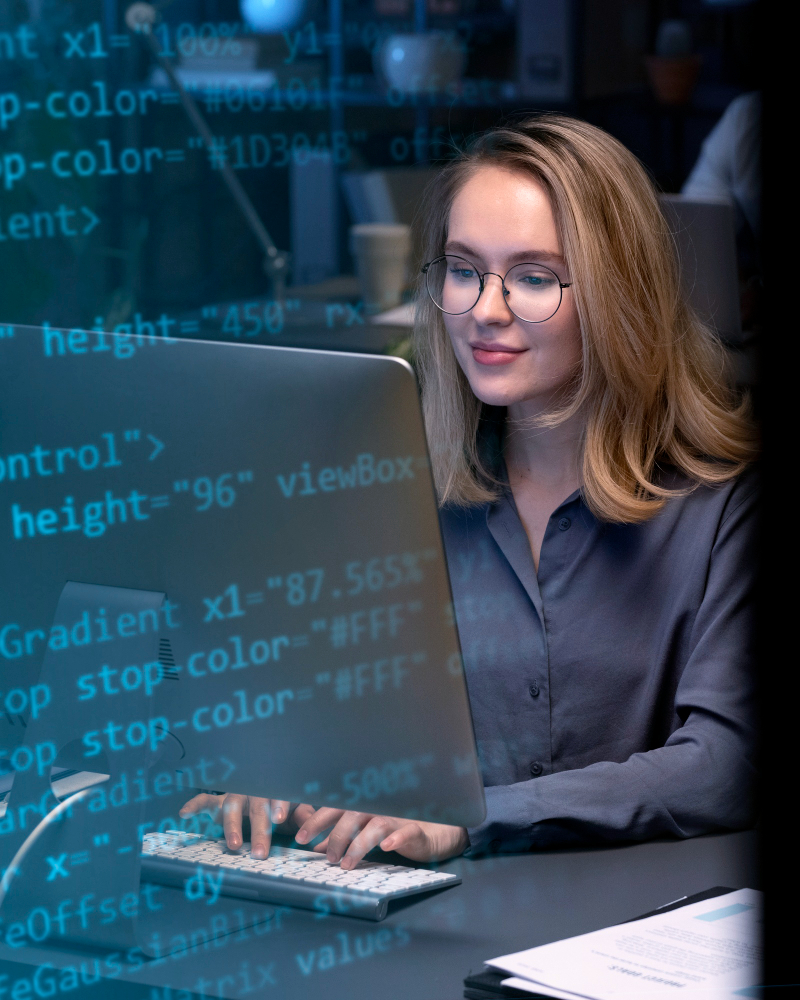
In this article, we delve into the fascinating world of optimizing string concatenation in Python code. As every experienced developer knows, the efficient manipulation of strings can significantly impact the performance of your code. Join us as we uncover the challenges faced when dealing with string concatenation and explore powerful techniques to boost your code’s execution time. By the end of this article, you’ll have a strong grasp on various optimization strategies, equipping you with the knowledge to enhance the speed and efficiency of your Python programs. So, let’s dive in and supercharge your string concatenation skills!
String
A string is a sequence of characters, like a word or a sentence. For example, “Hello, World!” is a string.
Concatenation
Concatenation is a fancy word for combining or joining things together. When we talk about string concatenation, we mean joining two or more strings to create a new, longer string.
Concept of String Concatenation:
Imagine you have two strings, let’s call them string1 and string2. String concatenation is the process of putting these two strings together to form a new, longer string.
In many programming languages, you can use the + (plus) operator to concatenate strings. Here’s a simple example in a fictional programming language:
string1 = “Hello, “
string2 = “World!”
result_string = string1 + string2
In this example, result_string will be “Hello, World!” because we joined string1 and string2 using the + operator.
Practical Example:
Let’s say you have a program that asks a user for their first name and last name. You can use string concatenation to create a full name:
first_name = input(“Enter your first name: “)
last_name = input(“Enter your last name: “)
full_name = first_name + ” ” + last_name
print(“Your full name is:”, full_name)
Here, the space between first_name and last_name is added using the string literal ” ” to ensure there’s a space between the first and last names in the full_name string.
Understanding String Concatenation
Understanding String Concatenation:String concatenation is the process of combining two or more strings into a single string. In Python, it is a common operation used in various scenarios, such as generating output messages, building URLs, or constructing complex data structures. While seemingly straightforward, understanding the intricacies of string concatenation is crucial for optimizing code performance.
At its core, string concatenation involves creating a new string by appending multiple strings together. However, it’s essential to be aware that strings are immutable objects in Python. This means that every time concatenation occurs, a new string object is created in memory. Consequently, if performed inefficiently or repeatedly within loops or functions, this can lead to unnecessary memory allocation and impact overall performance.
To effectively optimize string concatenation in Python code and enhance performance gains, it becomes imperative to delve deeper into the different methods available and their respective trade-offs. By exploring these techniques and understanding their nuances, we can unlock significant improvements in our code’s speed and efficiency.
1. Using the + Operator:
In Python, you can concatenate strings using the + operator. Here’s a simple example:
# Example 1: Using the + operator
first_name = “John”
last_name = “Doe”
full_name = first_name + ” ” + last_name
print(“Full Name:”, full_name)
In this example, we create two strings (first_name and last_name) and then use the + operator to concatenate them with a space in between, creating the full_name string.
2. Using the += Operator:
You can also use the += operator to concatenate and update a string in place. Here’s an example:
# Example 2: Using the += operator
message = “Hello, “
name = “Alice”
message += name
print(“Combined Message:”, message)
In this example, the += operator is used to add the name string to the end of the message string, updating the message variable in place.
3. Using the join() Method:
The join() method is another way to concatenate strings, especially when you have a list of strings. Here’s an example:
# Example 3: Using the join() method
words = [“This”, “is”, “a”, “sentence”]
sentence = ” “.join(words)
print(“Complete Sentence:”, sentence)
In this example, the join() method is used to concatenate the strings in the words list with a space in between, creating the sentence string.
Benefits of Using f-strings for String Concatenation
1.Readability:
F-strings make your code easy to read. When you’re mixing text and variables, f-strings allow you to directly include the variables within the string, making it clear what values you’re using without complicated syntax.
Example:
name = “Alice”
age = 20
print(f”Hello, {name}! You are {age} years old.”)
2.Simplicity:
F-strings simplify the process of combining different data types. You can effortlessly mix text and numbers without worrying about converting them to strings first.
Example:
item = “Apples”
quantity = 5
print(f”I bought {quantity} {item}.”)
3.Efficiency:
F-strings are faster and more efficient than other methods of string formatting in Python. This means your code runs smoothly and quickly, especially when dealing with a large amount of text or variables.
Example:
width = 10
height = 5
area = width * height
print(f”The area of the rectangle is {area} square units.”)
4.Less Room for Errors:
F-strings reduce the chances of making mistakes in your code. With traditional methods, you might forget to convert a variable to a string, leading to errors. F-strings handle this conversion for you.
Example:
x = 3
y = 4
print(f”The sum of {x} and {y} is {x + y}.”)
Profiling and Benchmarking String Concatenation
To truly optimize string concatenation in Python, it is crucial to profile and benchmark different approaches. Profiling allows us to identify the bottlenecks in our code, while benchmarking helps us compare the performance of various methods. By combining these techniques, we can gain valuable insights into the efficiency of different concatenation strategies.
During profiling, we meticulously examine our code’s execution time and resource usage. This process reveals the areas where string concatenation may be causing performance slowdowns. It enables us to pinpoint specific lines or functions that are taking longer than expected or consuming excessive memory. Armed with this knowledge, we can focus our optimization efforts on those critical sections.
Benchmarking takes profiling a step further by comparing the performance of multiple concatenation techniques under controlled conditions. By executing each method with a standardized test case and measuring their respective execution times, we can objectively determine which approach offers the best performance gains. This empirical data empowers us to make informed decisions regarding which method to adopt for optimal string concatenation.
Both profiling and benchmarking provide invaluable insights into how our code performs during string concatenation operations. Armed with this knowledge, we are equipped to identify inefficiencies and implement optimizations that boost overall performance significantly. By investing time in these processes, we can create Python code that not only meets but exceeds our expectations when it comes to string concatenation efficiency.
Techniques to Optimize String Concatenation in Python
Python offers several techniques to optimize string concatenation, ensuring efficient code performance. One powerful approach is to use the ‘join()’ method, which concatenates a list of strings with a specified delimiter. By creating a list of strings and joining them using ‘join()’, we avoid the overhead of repeatedly creating new string objects, resulting in faster execution.
Another technique is leveraging f-strings, introduced in Python 3.6. F-strings provide a concise and efficient way to format strings by embedding expressions inside curly braces {}. This not only enhances code readability but also improves performance compared to traditional string concatenation methods.
Furthermore, utilizing string formatting techniques like ‘%s’ or ‘{}’.format() can significantly optimize string concatenation. These methods allow placeholders for variables within the string and automatically replace them with their respective values. With proper usage, these formatting techniques contribute to more elegant and efficient code execution.
By employing these optimization techniques, developers can enhance the performance of their Python programs while maintaining clean and readable code. Embracing efficient coding practices not only boosts productivity but also contributes positively towards creating robust and high-performing applications.
Considerations for Large-scale String Concatenation
When dealing with large-scale string concatenation in Python, there are several important considerations to keep in mind. One of the main concerns is memory usage. As the size of the strings being concatenated increases, so does the memory required to store them. This can become a bottleneck and lead to inefficient performance.
To mitigate this issue, it is advisable to use alternative methods such as the ‘join()’ method or f-strings. The ‘join()’ method allows for concatenating a list of strings efficiently by utilizing a delimiter. This approach minimizes memory overhead by avoiding repeated string creation.
Another consideration is the choice of data structures used for storing intermediate results during concatenation. Using mutable data structures like lists or arrays can be more efficient than immutable ones such as tuples or strings since they allow for in-place modification and avoid unnecessary memory allocations.
Lastly, parallelization techniques can be explored to improve performance when dealing with large-scale string concatenation. By dividing the workload across multiple cores or machines, it is possible to reduce processing time significantly and achieve faster results.
Conclusion
In conclusion, optimizing string concatenation in Python can significantly improve the performance of your code, ensuring efficient memory usage and reducing execution time. By understanding the different techniques and approaches discussed in this article, you possess the knowledge to choose the most suitable method for your specific use case. Embracing these optimization strategies will not only enhance the overall efficiency of your Python programs but also empower you to tackle more complex tasks with confidence. So, go forth and harness the power of efficient string concatenation, unlocking new levels of performance and productivity in your Python projects.