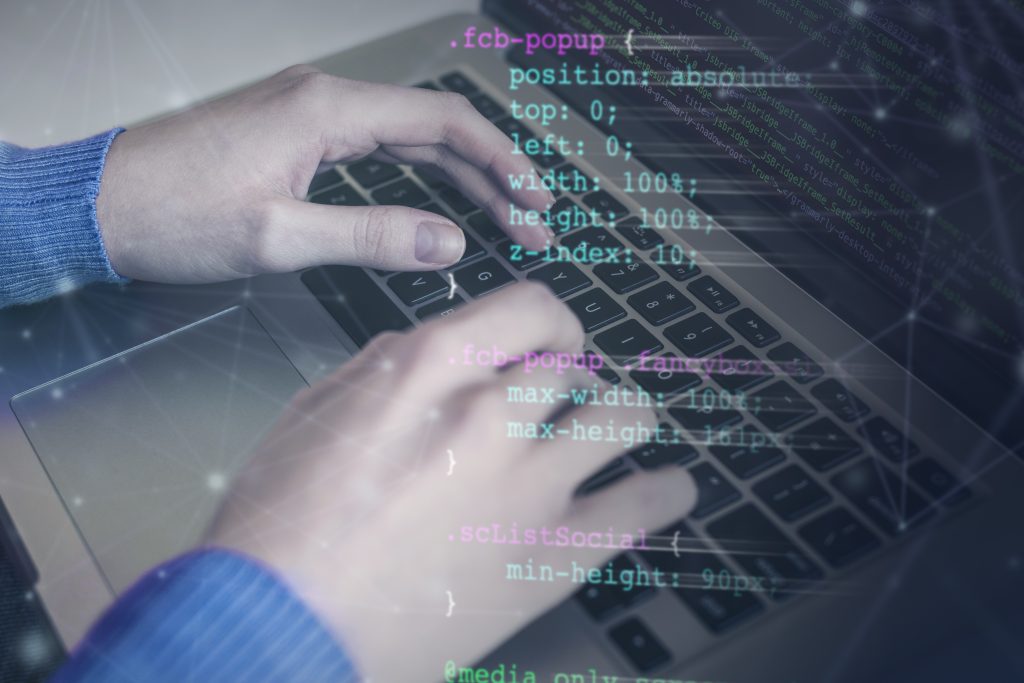
In programming, “n Number Summation” refers to the process of adding up a series of numbers, where ‘n’ represents the total count of numbers you want to add. This concept is essential in many applications, from calculating averages to solving complex mathematical problems.
For beginners, let’s start with a simple C program that sums up the first ‘n’ natural numbers. The formula for the sum of the first ‘n’ natural numbers is given by n⋅(n+1)/2
#include <stdio.h>
int main() {
int n, sum;
// Get user input for ‘n’
printf(“Enter the value of n: “);
scanf(“%d”, &n);
// Calculate the sum using the formula
sum = n * (n + 1) / 2;
// Display the result
printf(“Sum of the first %d natural numbers is %d.\n”, n, sum);
return 0;
}
Variables and Data Types in n Number Summation
In a C program, variables are like containers that hold data. Data types define the type of data that a variable can hold. Here’s an example:
#include <stdio.h>
int main() {
// Declare variables
int n; // ‘n’ will store the number of elements
int sum = 0; // ‘sum’ will store the total summation
// Get input from the user
printf(“Enter the number of elements: “);
scanf(“%d”, &n);
// Summation logic using a loop
for (int i = 1; i <= n; i++) {
sum += i; // Add ‘i’ to ‘sum’
}
// Display the result
printf(“Sum of first %d natural numbers is: %d\n”, n, sum);
return 0;
}
In this example:
- int n; declares a variable n of type integer to store the number of elements.
- int sum = 0; declares a variable sum and initializes it to 0. It will store the total summation.
- The scanf function is used to take input from the user.
- The for loop is used to iterate through numbers from 1 to n and add them to the sum.
Example Explanation:
Suppose the user enters 5 as the number of elements. The program then calculates the sum of the first 5 natural numbers (1 + 2 + 3 + 4 + 5) and prints the result, which is 15.
This program illustrates the use of variables (n and sum) and the importance of choosing the right data type (int for whole numbers) to perform a simple summation. Understanding these concepts is fundamental when working with data and performing calculations in programming.
The Role of Loops in n Number Summation
Implementing n Number Summation using For Loop:
the role of loops in the summation of numbers in a C program, specifically using a for loop. Understanding loops is crucial for efficiently performing repetitive tasks, such as summing up a series of numbers.
#include <stdio.h>
int main() {
// Declare variables
int n, sum = 0;
// Prompt user for input
printf(“Enter a positive integer n: “);
scanf(“%d”, &n);
// Check if n is a positive integer
if (n < 1) {
printf(“Please enter a positive integer.\n”);
return 1; // Exit program with an error code
}
// Using a for loop to calculate the sum
for (int i = 1; i <= n; i++) {
sum += i; // Add the current value of i to the sum
}
// Display the result
printf(“Sum of the first %d natural numbers = %d\n”, n, sum);
return 0; // Exit program successfully
}
Now, let’s break down the code and explain it in a way that a college student can understand:
- Initialization of Variables:
- int n, sum = 0;: We declare two variables, n to store the user input (the limit of summation), and sum to store the cumulative sum.
- User Input:
- We prompt the user to enter a positive integer n using printf and scanf.
- Input Validation:
- We check if the entered value of n is a positive integer. If not, we display an error message and exit the program.
- For Loop:
- for (int i = 1; i <= n; i++): This is a for loop that initializes a loop control variable i to 1. It continues as long as i is less than or equal to n, and after each iteration, it increments i by 1.
- sum += i;: In each iteration, we add the current value of i to the sum. This is the crucial step that accumulates the sum of the numbers.
- Display Result:
- We print the calculated sum using printf.
In summary, the for loop efficiently handles the repetitive task of adding numbers from 1 to n, making the code concise and easy to understand. It’s a fundamental concept in programming, and mastering loops is essential for writing efficient and scalable code.
Implementing n Number Summation using While Loop
Let’s create a simple C program to implement the summation of the first n natural numbers using a while loop. The concept here is to initialize a variable to store the sum, then use a while loop to iterate through the numbers from 1 to n and add them to the sum.
#include <stdio.h>
int main() {
// Declare variables
int n, i, sum;
// Initialize sum to 0
sum = 0;
// Ask the user for input
printf(“Enter a positive integer n: “);
scanf(“%d”, &n);
// Validate if n is a positive integer
if (n <= 0) {
printf(“Please enter a positive integer.\n”);
return 1; // Exit the program with an error code
}
// Calculate the sum using a while loop
i = 1; // Start from the first natural number
while (i <= n) {
sum = sum + i; // Add the current number to the sum
i++; // Move to the next number
}
// Display the result
printf(“The sum of the first %d natural numbers is: %d\n”, n, sum);
return 0; // Exit the program successfully
}
Explanation:
1.Declaration and Initialization: We declare three variables – n to store the user input, i to iterate through numbers, and sum to store the sum of numbers. We initialize sum to 0.
- 2.User Input: We ask the user to enter a positive integer n.
- Validation: We check if n is a positive integer. If not, we print an error message and exit the program.
- 3.While Loop: We use a while loop to iterate from 1 to n. In each iteration, we add the current number (i) to the sum.
- 4.Display Result: Finally, we print the sum of the first n natural numbers.
Implementing n Number Summation using Do-While Loop
Let’s create a simple C program that implements the summation of n numbers using a do-while loop. The program will prompt the user to enter the value of n, and then it will ask the user to enter n numbers for summation. Finally, it will display the sum of those n numbers.
Here’s the C program:
#include <stdio.h>
int main() {
// Declare variables
int n, i = 1, num, sum = 0;
// Get the value of n from the user
printf(“Enter the value of n: “);
scanf(“%d”, &n);
// Check if n is greater than 0
if (n <= 0) {
printf(“Please enter a positive value for n.\n”);
return 1; // Exit the program with an error code
}
// Prompt the user to enter n numbers
printf(“Enter %d numbers:\n”, n);
// Use a do-while loop to get n numbers and calculate the sum
do {
printf(“Enter number %d: “, i);
scanf(“%d”, &num);
// Add the entered number to the sum
sum += num;
// Increment the counter
i++;
} while (i <= n); // Continue the loop until i is greater than n
// Display the sum
printf(“The sum of the entered %d numbers is: %d\n”, n, sum);
return 0; // Exit the program successfully
}
Explanation:
- 1.We declare variables to store the user input (n, num), a counter (i), and the sum of the numbers.
- 2.We prompt the user to enter the value of n.
- 3.We use a do-while loop to repeatedly ask the user to enter n numbers. The loop continues until the counter i is greater than n.
- 4.Inside the loop, we prompt the user to enter a number, add it to the sum, and increment the counter.
- 5.After the loop, we display the sum of the entered numbers.
- 6.We include a check to ensure that the value of n is positive.
Real-Life Applications and Use Cases of n Number Summation in C Programming
One of the remarkable aspects of n number summation in C programming is its versatility, which gives rise to numerous real-life applications. For instance, financial institutions heavily rely on this concept to calculate interest rates, compound interests, and even mortgage payments. The ability to accurately compute the sum of a series of numbers allows banks and loan providers to streamline their operations and provide customers with precise information regarding their repayment schedules.