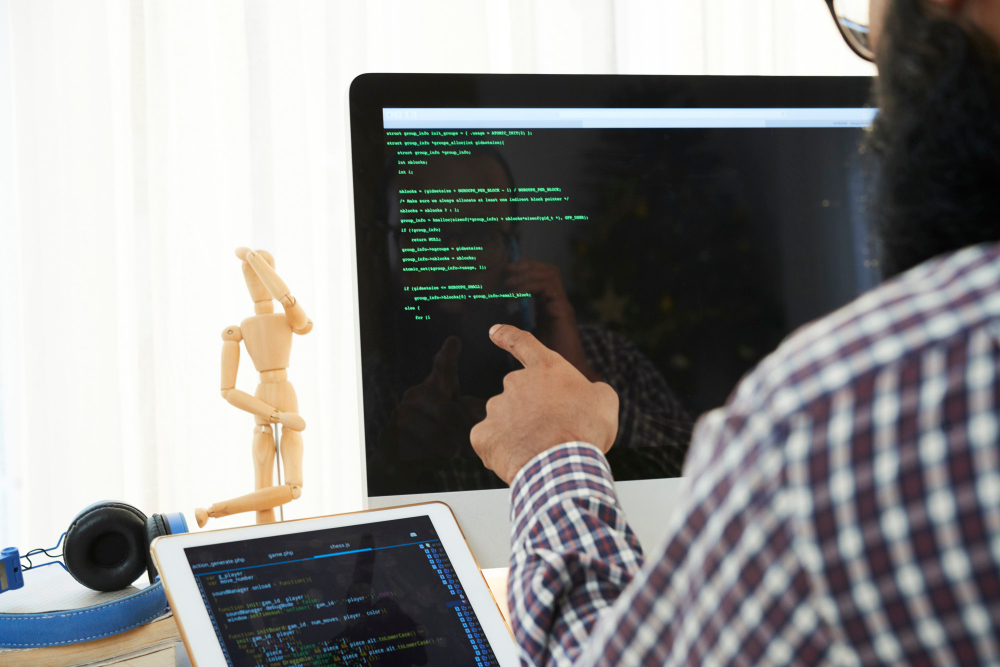
In this article, we delve into the realm of Python programming to equip you with the powerful techniques of string combining. Say goodbye to convoluted code and hello to streamlined efficiency. Whether you’re a seasoned coder or just starting out, we’ve got you covered. Brace yourself for invaluable insights and practical examples that will revolutionize your Python programs. Prepare to witness the seamless blending of strings, unlocking a whole new level of program elegance. Your quest for enhanced Python programs starts here. Get ready to unleash the potential of your code!
Fancier Output Formatting
• To use formatted string literals, begin a string with f or F before the opening quotation mark or triple quotation mark. Inside this string, you can write a Python expression between { and } characters that can refer to variables or literal values.
>>> year = 2016 ; event = ‘Referendum’
>>> f’Results of the {year} {event}’
‘Results of the 2016 Referendum’
• The str.format() method of strings requires more manual effort. You’ll still use { and } to mark where a variable will be substituted and can provide detailed formatting directives, but you’ll also need to provide the information to be formatted.
>>> yes_votes = 42_572_654 ; no_votes = 43_132_495
>>> percentage = yes_votes/(yes_votes+no_votes)
>>> ‘{:-9} YES votes {:2.2%}’.format(yes_votes, percentage)
‘ 42572654 YES votes 49.67%’
• Finally, you can do all the string handling yourself by using string slicing and concatenation operations to create any layout you can imagine. The string type has some methods that perform useful operations for padding strings to a given column width.
When you don’t need fancy output but just want a quick display of some variables for debugging purposes, you can convert any value to a string with the repr() or str() functions.
The str() function is meant to return representations of values which are fairly human-readable, while repr() is meant to generate representations which can be read by the interpreter (or will force a SyntaxError if there is no equivalent syntax). For objects which don’t have a particular representation for human consumption, str() will return the same value as repr().
Many values, such as numbers or structures like lists and dictionaries, have the same representation using either function. Strings, in particular, have two distinct representations. Some examples:
5 and efficient code that not only functions flawlessly but also leaves a lasting positive impact on its users.
>>> s = ‘Hello, world.’
>>> str(s)
‘Hello, world.’
>>> repr(s)
“‘Hello, world.'”
>>> str(1/7)
‘0.14285714285714285’
>>> x = 10 * 3.25
>>> y = 200 * 200
>>> s = ‘The value of x is ‘ + repr(x) + ‘, and y is ‘ + repr(y) + ‘…’
>>> print(s)
The value of x is 32.5, and y is 40000…
>>> # The repr() of a string adds string quotes and backslashes:
… hello = ‘hello, world\n’
>>> hellos = repr(hello)
>>> print(hellos)
‘hello, world\n’
>>> # The argument to repr() may be any Python object:
… repr((x, y, (‘spam’, ‘eggs’)))
“(32.5, 40000, (‘spam’, ‘eggs’))”
The string module contains a Template class that offers yet another way to substitute values into strings, using placeholders like $x and replacing them with values from a dictionary, but offers much less control of the formatting.
Formatted String Literals
Formatted string literals (also called f-strings for short) let you include the value of Python expressions inside a string by prefixing the string with f or F and writing expressions as {expression}.
An optional format specifier can follow the expression. This allows greater control over how the value is formatted. The following example rounds pi to three places after the decimal:
>>> import math
>>> print(f’The value of pi is approximately {math.pi:.3f}.’)
Passing an integer after the ‘:’ will cause that field to be a minimum number of characters wide. This is useful for making columns line up.
>>> table = {‘Sjoerd’: 4127, ‘Jack’: 4098, ‘Dcab’: 7678}
>>> for name, phone in table.items():
… print(f'{name:10} ==> {phone:10d}’)
…
Sjoerd ==> 4127
Jack ==> 4098
Dcab ==> 7678
Other modifiers can be used to convert the value before it is formatted. ‘!a’ applies ascii(), ‘!s’ applies str(), and ‘!r’ applies repr():
>>> animals = ‘eels’
>>> print(f’My hovercraft is full of {animals}.’)
My hovercraft is full of eels.
>>> print(‘My hovercraft is full of {animals !r}.’)
My hovercraft is full of ‘eels’.
The String format() Method
Basic usage of the str.format() method looks like this:
>>> print(‘We are the {} who say “{}!”‘.format(‘knights’, ‘Ni’))
We are the knights who say “Ni!”
The brackets and characters within them (called format fields) are replaced with the objects passed into the str.format() method. A number in the brackets can be used to refer to the position of the object passed into the str.format() method.
>>> print(‘{0} and {1}’.format(‘spam’, ‘eggs’))
spam and eggs
>>> print(‘{1} and {0}’.format(‘spam’, ‘eggs’))
eggs and spam
If keyword arguments are used in the str.format() method, their values are referred to by using the name of the argument.
>>> print(‘This {food} is {adjective}.’.format( … food=’spam’, adjective=’absolutely horrible’))
This spam is absolutely horrible.
Positional and keyword arguments can be arbitrarily combined:
>>> print(‘The story of {0}, {1}, and {other}.’.format(‘Bill’, ‘Manfred’, other=’Georg’))
The story of Bill, Manfred, and Georg.
If you have a really long format string that you don’t want to split up, it would be nice if you could reference the variables to be formatted by name instead of by position. This can be done by simply passing the dict and using square brackets ‘[]’ to access the keys
>>> table = {‘Sjoerd’: 4127, ‘Jack’: 4098, ‘Dcab’: 8637678}
>>> print(‘Jack: {0[Jack]:d}; Sjoerd: {0[Sjoerd]:d}; ‘ … ‘Dcab: {0[Dcab]:d}’.format(table))
Jack: 4098; Sjoerd: 4127; Dcab: 8637678
This could also be done by passing the table as keyword arguments with the ‘**’ notation.
>>> table = {‘Sjoerd’: 4127, ‘Jack’: 4098, ‘Dcab’: 8637678}
>>> print(‘Jack: {Jack:d}; Sjoerd: {Sjoerd:d}; Dcab: {Dcab:d}’.format(**table))
Jack: 4098; Sjoerd: 4127; Dcab: 8637678
This is particularly useful in combination with the built-in function vars(), which returns a dictionary containing all local variables.
Utilizing string concatenation for basic string combining
String concatenation is a fundamental technique for combining multiple strings into a single cohesive unit. In Python, this can be achieved simply by using the ‘+’ operator to concatenate two or more strings together. For example, if we have two strings, ‘Hello’ and ‘world’, we can combine them using the ‘+’ operator like this: ‘Hello’ + ‘world’, which would result in the string ‘Helloworld’.
This basic string combining technique allows us to create complex and meaningful sentences or phrases by joining together different words or phrases. It is particularly useful when we have static strings that need to be combined, without any dynamic elements involved. By harnessing the power of string concatenation, we can build informative output messages or construct well-formed queries for database operations.
Remember that when utilizing string concatenation, it’s essential to pay attention to proper spacing and punctuation to ensure readability and coherence of the resulting concatenated string. Moreover, by adopting efficient coding practices like using formatted strings or employing external libraries for advanced string manipulation tasks, we can further enhance our Python programs and promote code reusability and maintainability
Incorporating string interpolation for dynamic and efficient string combining
String interpolation is a powerful technique in Python that allows for the seamless integration of variables within string literals. By using curly brackets {} as placeholders and the format() method, we can dynamically combine strings with variable values.
This approach offers a concise and readable solution, enabling us to easily incorporate variable values into our strings without the need for explicit concatenation or complex formatting codes. It promotes code reusability and enhances readability by keeping the logic separate from the presentation.
Moreover, string interpolation provides flexibility by accepting a wide variety of data types, including integers, floats, booleans, and even custom objects. With this versatility, we can effortlessly create informative messages tailored to specific situations or users. By leveraging string interpolation effectively, we can elevate the quality of our Python programs while maintaining simplicity and elegance.
So why settle for static strings when you can inject life into your programs? Embrace the power of string interpolation in Python to unlock dynamic and efficient string combining capabilities that will enhance both your code’s functionality and its aesthetic appeal.
Implementing string interpolation with the % operator
The % operator in Python provides a powerful and flexible way to perform string interpolation, enabling dynamic value insertion within a string. This technique allows you to create more readable and efficient code by eliminating the need for cumbersome concatenation or formatting operations.
To use the % operator for string interpolation, you simply include placeholders in your string using the %s symbol. These placeholders act as markers where values can be inserted later. For example, consider the following code snippet:
name = “Alice”
age = 25
message = “My name is %s and I am %d years old.” % (name, age)
In this example, the variables ‘name’ and ‘age’ are inserted into the ‘message’ string using the % operator. The ‘%s’ placeholder is used for inserting a string value (in this case, ‘name’), while ‘%d’ is used for inserting an integer value (in this case, ‘age’).
Using the % operator offers not only simplicity but also efficiency. It eliminates unnecessary concatenation operations by directly incorporating values into strings without additional steps. By adopting this approach, your code becomes more concise and easier to maintain.
So, embrace the power of the % operator in Python to enhance your string combining techniques. Its versatility enables you to effortlessly incorporate dynamic values into your strings with elegance and efficiency, making your programs more attractive and robust.
Combining strings with the help of external libraries and modules
In the world of Python, there is a vast ecosystem of external libraries and modules that can greatly enhance your string combining capabilities. One such library is the popular ‘f-string’ module, which allows for more concise and readable string interpolation. With f-strings, you can embed expressions directly within curly braces, making it easier than ever to combine variables and strings effortlessly.
Another powerful tool at your disposal is the ‘format’ method provided by Python’s built-in ‘string’ module. This method allows you to define placeholders in a string and then substitute them with corresponding values at runtime. It provides a flexible and versatile approach to string combining, allowing for easy formatting of numbers, dates, and more complex data structures.
Lastly, if you’re looking for advanced functionality or specialized formatting options, consider leveraging external libraries like ‘textwrap’ or ‘prettytable’. The ‘textwrap’ library offers utilities for wrapping and aligning text within strings, while ‘prettytable’ provides an elegant way to create well-formatted tables from data. These libraries not only simplify your code but also add a touch of professionalism to your program’s output.
By exploring these external libraries and modules in Python’s rich ecosystem, you can elevate your string combining techniques to new heights. Whether it’s achieving cleaner syntax with f-strings or creating beautifully formatted tables using specialized libraries, these tools empower you to deliver polished and impressive results while saving time and effort in the process
Conclusion
In conclusion, mastering effective string combining techniques can greatly enhance the functionality and efficiency of your Python programs. By understanding the different methods available, such as string concatenation, interpolation with the % operator, and utilizing external libraries and modules, you can optimize your code and achieve desired results with elegance. With these powerful tools at your disposal, you have the ability to create dynamic and expressive strings that will captivate both yourself and your audience.