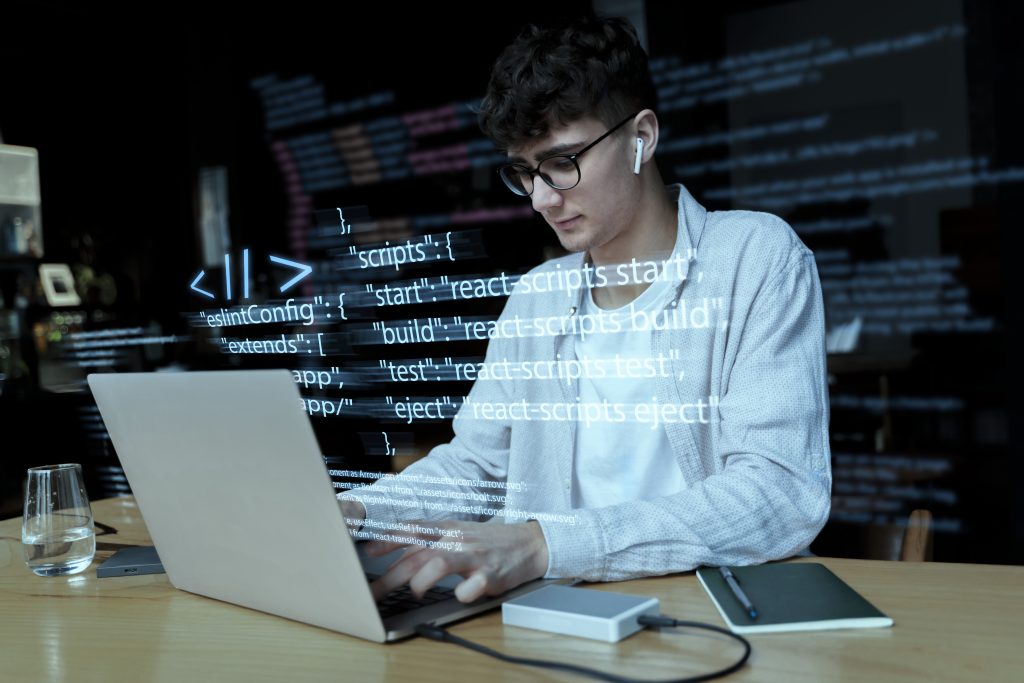
Understanding Singly Linked Lists
Linked lists are a fundamental data structure in computer science, consisting of a sequence of nodes connected by pointers. In the case of a singly linked list, each node contains both data and a reference to the next node in the sequence. This simple yet powerful structure offers flexibility and efficiency for dynamic storage.
Imagine each node as a unique character in an enthralling story, where they hold vital information while pointing towards the next intriguing chapter. Like turning pages, traversing a singly linked list allows us to explore its contents sequentially, unveiling the narrative it holds within.
Singly linked lists offer several advantages over other data structures. They provide constant time complexity for insertion and deletion at either end of the list, making them ideal for scenarios where frequent modifications are required. Furthermore, their dynamic nature enables efficient memory utilization as nodes can be easily added or removed on-demand.
So let’s embark on this journey together as we unravel the intricacies of mastering operations on singly linked lists in Java. By understanding their foundations and inner workings, we will unlock the potential to create elegant and efficient solutions that breathe life into our code.
Insertion Techniques
One of the fundamental operations in working with singly linked lists in Java is insertion. Inserting new nodes at various positions within the linked list allows for dynamic updates and efficient data management. There are three primary insertion techniques to be explored: insertion at the beginning, insertion at the end, and insertion at a specific position within the linked list.To start with, inserting a node at the beginning of a linked list involves creating a new node and updating its next reference to point to the current head of the list. The head pointer is then updated to point to this newly inserted node. This technique provides constant time complexity and ensures quick access to newly added elements.
Moving on, inserting a node at the end of a linked list requires traversing through all existing nodes until reaching the last one. Once there, a new node is created and its reference is assigned as null since it will become the last element in the list. The previous last node’s next reference is then updated to point towards this new node, making it part of the list.
Lastly, inserting a node at a specific position within the linked list involves traversing through nodes until reaching the desired position – either by counting nodes or following references based on indices – before performing similar steps as in regular insertion. This technique allows for precise control over where an element should be inserted within an ordered or sorted linked list.
Mastering these insertion techniques opens up possibilities for efficient data manipulation and organization within singly linked lists in Java, providing programmers with powerful tools for handling dynamic data structures with ease and elegance.
Deletion Techniques
Deleting nodes from a singly linked list is an essential operation that ensures the integrity and efficiency of the data structure. There are three primary deletion techniques: deletion at the beginning, deletion at the end, and deletion of a specific node within the linked list.
When deleting a node from the beginning of a singly linked list, we simply update the head pointer to point to the next node, effectively skipping over the first node. This operation is efficient and guarantees constant time complexity. It allows for quick removal of unwanted elements while preserving the order of the remaining nodes.
In contrast, when deleting a node from the end of a singly linked list, we need to traverse through all its elements until we reach the second-to-last node. We then update its next reference to null, effectively removing it from our data structure. Although this technique requires linear time complexity due to traversing, it still offers an efficient approach for maintaining list consistency.
Finally, deleting a specific node within a linked list involves locating its position by traversing through each element until finding its predecessor node. We then adjust their reference pointers accordingly to bypass and remove our target node. While this technique may require extra operations compared to other deletions, it grants us flexibility in removing any desired element within our data structure.
By mastering these deletion techniques in Java’s implementation of singly linked lists, you will gain control over managing your data efficiently and maintaining logical consistency throughout your programs
Traversal Techniques
Traversing a singly linked list is an essential operation that allows us to access and process each element of the list sequentially. It forms the backbone of many algorithms and data structures. In Java, there are several approaches to traverse a linked list, each with its own advantages and use cases.One common technique is the iterative approach, where we start from the head of the linked list and move through each node until we reach the end. During traversal, we can perform various operations on each node, such as printing its value or modifying its data. This technique provides a straightforward way to access elements in a predetermined order.
Another traversal technique involves using recursion. By employing a recursive function, we can elegantly traverse through the linked list by moving to the next node with each recursive call until we reach the end. This approach offers concise code but may have limitations concerning large datasets due to potential stack overflow issues.
To optimize traversal speed in certain scenarios, we can implement techniques like caching or memoization. These methods involve storing previously accessed values in memory for faster retrieval later on. By employing such techniques judiciously, we can enhance performance and efficiency when traversing lengthy linked lists.
Overall, mastering traversal techniques equips us with powerful tools for efficiently navigating singly linked lists in Java. Whether using iterative or recursive approaches, or even leveraging optimization methods like caching, these techniques empower developers to process data effectively and unlock vast possibilities for solving complex problems with elegance and finesse
Insertion at the Beginning of the Linked List
When it comes to inserting a new node at the beginning of a singly linked list in Java, elegance and efficiency are key. To accomplish this, we need to create a new node with the desired data and make it point to the current head node. By updating the head reference to point to our newly created node, we seamlessly insert it at the beginning.
This operation is particularly useful when we want to prioritize a new element over existing ones. Imagine you have a task management application where each task represents a node in your linked list. With insertion at the beginning, you can effortlessly add high-priority tasks, ensuring they are addressed promptly and efficiently.
Implementing this insertion technique involves just a few steps: creating a new node, setting its data value, pointing the next reference of this new node to the current head of the list, and finally updating the head reference itself. By doing so, we seamlessly integrate our new element into our linked list structure without disturbing its existing nodes. This efficient approach guarantees that no matter how long your linked list becomes or how many elements it holds, adding an item at its beginning remains swift and hassle-free
Insertion at the End of the Linked List
To add a new node at the end of a singly linked list in Java, we follow a simple yet effective approach. First, we need to identify if the list is empty or not. If it is empty, we can directly create a new node and make it the head of our list. However, if the list already contains elements, we traverse through it until we reach the last node. Then, we create a new node and make it the next node of our last element.Adding an element to the end of a linked list is like extending one’s hand in friendship – both simple and meaningful. It symbolizes inclusiveness by expanding an existing community with open arms. Similarly, when we insert at the end of a linked list, we contribute to its growth and continuity. This technique allows us to seamlessly expand our data structure while preserving its existing connections, fostering a sense of harmony within our code.
Insertion at a Specific Position in the Linked List
In the fascinating realm of Singly Linked Lists, inserting a node at a specific position adds an element of challenge and excitement. This operation allows us to precisely place our desired node within the list, expanding our control over its structure. With Java as our trusted companion, we embark on this journey with confidence.
To accomplish this feat, we navigate through the linked list until we reach the desired position. Our diligent traversal is guided by the pointers that link each node together, leading us closer to our destination. Once positioned correctly, we create a new node and skillfully adjust the pointers to incorporate it seamlessly into the list.
This process empowers us to establish order and harmony within our linked list universe. By precisely placing nodes at specific positions, we shape our data structure with intention and purpose. Through this mastery of insertion techniques, we create a symphony of interconnected nodes that harmonize beautifully to fulfill their collective purpose.
Deletion at the Beginning of the Linked List
To master the art of deleting nodes from the beginning of a singly linked list in Java, we need to understand the underlying process involved. The first step is to identify and isolate the head node, which represents the starting point of our linked list. By reassigning the head’s next pointer to its subsequent node, we seamlessly detach it from our structure. This process not only frees up memory but also preserves the integrity of our data structure.Imagine this: you are a conductor leading an orchestra, and the linked list is your symphony. As you carefully remove a musician from their designated spot in harmony with others, you maintain impeccable synchronization in your composition. With each deletion at the beginning, you create room for new melodies to flourish while keeping the rhythm intact. Thus, by mastering this technique, you orchestrate a harmonious balance between preservation and progress within your linked list symphony.
Practice makes perfect! By embracing these deletion techniques at the beginning of a singly linked list in Java, you become an agile conductor capable of maintaining a finely tuned symphony that evolves with grace and efficiency. Through thoughtful deletions and purposeful rearrangements, you ensure that your musical masterpiece thrives – just as your linked list will continue to flourish with every deletion at its very inception.
Deletion at the End of the Linked List
Deleting a node at the end of a singly linked list is an essential operation for efficiently managing the list’s contents. To accomplish this, we must traverse the entire list until we reach the second-to-last node, also known as the penultimate node. By doing so, we can then update its next reference to null, effectively severing its connection to the last node and removing it from the list.
Thought-Provoking Content:
The process of deleting a node at the end of a linked list teaches us an important lesson about letting go. Just as we remove unnecessary nodes from our data structure to enhance its efficiency, sometimes in life, we need to let go of things that no longer serve us. By embracing this concept, we create room for growth and allow new opportunities to enter our lives.
Optimistic Spin:
Though deletion may seem like an act of loss or removal, it actually contributes positively to our linked list by streamlining its structure and optimizing its performance. Similarly, in life, letting go of what no longer serves us can lead to personal growth and pave the way for exciting new adventures
Deletion of a Specific Node in the Linked List
When it comes to removing a particular node from a singly linked list in Java, there are a few essential steps to follow. First, we need to locate the node that we want to delete. This can be achieved by traversing through the linked list until we find the desired node or reach the end of the list. Once identified, we must update the pointers accordingly to link the previous node with the next node, effectively skipping over and removing the targeted node.Although deleting a specific node may seem daunting at first, fear not! With Java’s vast array of built-in functions and logical operators, this task becomes relatively straightforward. By carefully adjusting pointers and updating references, we can seamlessly remove nodes from our linked list without compromising its integrity.
Traversing the Linked List
Traversing the Linked List:As we delve into the realm of traversing a singly linked list in Java, we embark on a journey filled with discovery and enlightenment. Picture yourself as an adventurous explorer, venturing through each node of the list, unraveling its secrets. With each step, you uncover a world of possibilities, where data comes alive and patterns emerge.
Indeed, traversing a linked list in Java allows us to unlock the potential hidden within its structure. It grants us access to a world that intertwines logic and creativity – where code becomes artistry and every node holds a story waiting to be told.