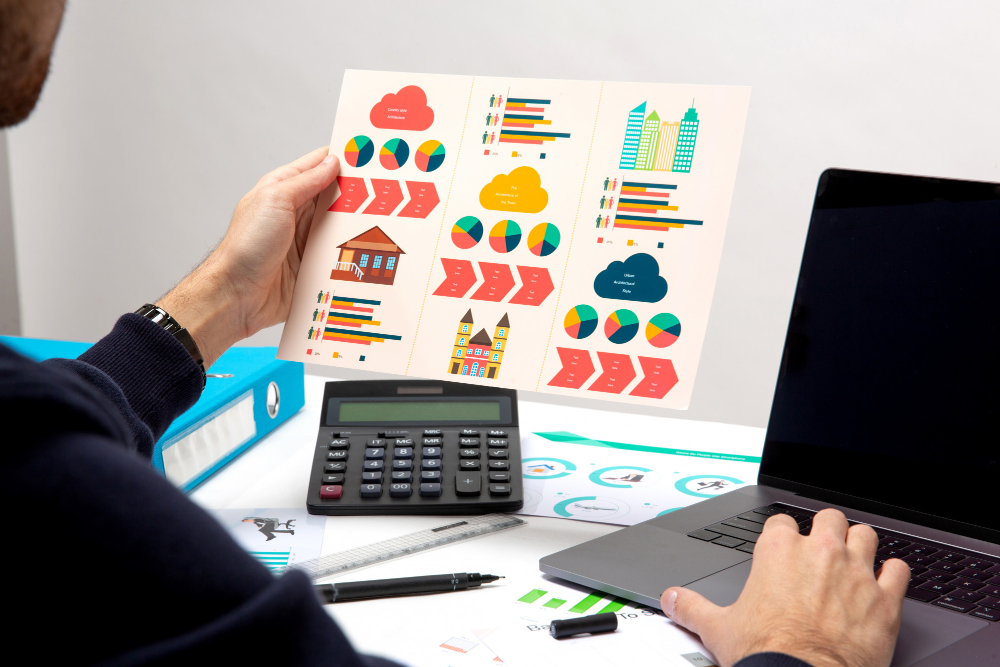
In this article, we delve into the captivating journey of bubble sorting, uncovering its humble beginnings and its evolution into the modern implementations we see today. As we explore the fascinating history behind this algorithm, brace yourself for a deep understanding of the problem it tackles and the journey it undertakes. Expect to be captivated by the milestones achieved and the innovations sprouting from this simple yet powerful sorting technique. Rest assured, this exploration promises to unlock insights that every reader craves – from novice programmers seeking foundational knowledge to seasoned developers yearning for a fresh perspective. So, fasten your seatbelts as we embark on a thought-provoking exploration into the remarkable evolution of bubble sorting.
What is a Sorting Algorithm?
In computer science, a sorting algorithm is a step-by-step procedure for rearranging the elements of a collection in a specific order. Sorting is a fundamental operation used in various applications, such as searching, data analysis, and information retrieval. One of the basic sorting algorithms that is often introduced early in computer science courses is the Bubble Sort algorithm.
Bubble Sort Overview:
Bubble Sort is a straightforward and easy-to-understand sorting algorithm. It works by repeatedly stepping through the list of elements, comparing adjacent items, and swapping them if they are in the wrong order. The pass through the list is repeated until the entire list is sorted.
Let’s break down the Bubble Sort algorithm into simple steps:
- Comparing and Swapping:
- Start from the beginning of the list.
- Compare the first two elements.
- If the first element is greater than the second, swap them; otherwise, leave them as they are.
- Move to the next pair of elements and repeat the process until you reach the end of the list.
- One Pass through the List:
- After the first pass, the largest element will be at the end of the list.
- Repeat the process for the remaining elements, excluding the last one since it’s already sorted.
- Repeat Until Sorted:
- Continue these passes through the list until no more swaps are needed, indicating that the entire list is sorted.
Let’s illustrate the Bubble Sort algorithm with a simple example:
Example:
Consider the following list of integers: 5, 2, 9, 1, 5, 6.
Step 1: Initial List
[5, 2, 9, 1, 5, 6]
Step 2: First Pass
[2, 5, 1, 5, 6, 9] (Swapped 5 and 2)
Step 3: Second Pass
[2, 1, 5, 5, 6, 9] (Swapped 5 and 1)
Step 4: Third Pass
[1, 2, 5, 5, 6, 9] (No swaps needed)
The list is now sorted, and the algorithm terminates.
Let us write a simple program for Bubble Algorithm
def bubble_sort(arr):
“””
Bubble Sort implementation in Python
Parameters:
arr (list): List of elements to be sorted
Returns:
list: Sorted list
“””
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already sorted, so we don’t need to check them
for j in range(0, n – i – 1):
# Swap if the element found is greater than the next element
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
# Example usage:
if __name__ == “__main__”:
# Input list to be sorted
my_list = [64, 34, 25, 12, 22, 11, 90]
print(“Original List:”, my_list)
# Applying Bubble Sort
bubble_sort(my_list)
print(“Sorted List:”, my_list)
Let’s break down the program:
- Function Definition:
- The bubble_sort function takes a list arr as input and sorts it using the Bubble Sort algorithm.
- Outer Loop (for i in range(n)):
- The outer loop iterates through each element of the list.
- The loop variable i represents the number of passes through the list.
- Inner Loop (for j in range(0, n – i – 1)):
- The inner loop compares adjacent elements and swaps them if they are in the wrong order.
- The loop variable j represents the index of the current element being compared.
- Swap Condition (if arr[j] > arr[j + 1]):
- If the current element is greater than the next element, a swap is performed.
- This ensures that the larger elements “bubble up” to their correct positions.
- Example Usage:
- An example list (my_list) is provided for demonstration.
- The original list is printed, the Bubble Sort algorithm is applied, and the sorted list is printed.
To better understand, consider the example list [64, 34, 25, 12, 22, 11, 90]:
- Pass 1:
- Comparisons: 64 vs. 34, 34 vs. 25, 25 vs. 12, 12 vs. 22, 22 vs. 11, 11 vs. 90 (swaps occur)
- Result: [34, 25, 12, 22, 11, 64, 90]
- Pass 2:
- Comparisons: 34 vs. 25, 25 vs. 12, 12 vs. 22, 22 vs. 11 (swaps occur)
- Result: [25, 12, 22, 11, 34, 64, 90]
- Pass 3:
- Comparisons: 25 vs. 12, 12 vs. 22, 22 vs. 11 (swaps occur)
- Result: [12, 22, 11, 25, 34, 64, 90]
- Pass 4:
- Comparisons: 12 vs. 22, 22 vs. 11 (swaps occur)
- Result: [12, 11, 22, 25, 34, 64, 90]
- Passes 5-6:
- Further comparisons and swaps until the list is fully sorted.
Efficiency and Time Complexity:
Bubble Sort is easy to understand, but it may not be the most efficient sorting algorithm for large datasets. The time complexity of Bubble Sort is O(n^2), where n is the number of elements in the list. This means that as the number of elements increases, the time taken to sort the list grows quadratically.
Despite its simplicity, Bubble Sort is often not the algorithm of choice for large datasets due to its inefficiency. However, it serves as a great introductory algorithm to help you grasp the fundamental concepts of sorting.
Advantages:
- Simple and easy to understand.
- Minimal space complexity (requires only a constant amount of additional memory).
Disadvantages:
- Inefficient for large datasets.
- Quadratic time complexity makes it impractical for large-scale applications.
Bubble Sorting Variants
1. Bubble Sort: A Quick Recap
Before we dive into the variants, let’s refresh our memory on the classic Bubble Sort:
- Basic Steps:
- Compare adjacent elements in the array.
- If they are in the wrong order, swap them.
- Continue this process until no more swaps are needed, indicating the array is sorted.
- Time Complexity: O(n^2) in the worst case.
Now, let’s explore some intriguing variants:
2. Optimized Bubble Sort:
This variant aims to improve the basic Bubble Sort by introducing a mechanism to detect whether any swaps were made during a pass through the array. If no swaps occurred, the algorithm concludes that the array is already sorted and terminates, saving unnecessary iterations.
Example:
def optimized_bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n – i – 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
swapped = True
# If no two elements were swapped, array is already sorted
if not swapped:
break
3. Recursive Bubble Sort:
In this variant, we leverage the power of recursion to implement Bubble Sort. The basic idea remains the same, but instead of using nested loops, we call the function recursively.
Example:
def recursive_bubble_sort(arr, n=None):
if n is None:
n = len(arr)
if n == 1:
return
for i in range(n – 1):
if arr[i] > arr[i + 1]:
arr[i], arr[i + 1] = arr[i + 1], arr[i]
recursive_bubble_sort(arr, n – 1)
4. Cocktail Shaker Sort (Bidirectional Bubble Sort):
This variant extends the idea of Bubble Sort by allowing the algorithm to move in both directions through the array. It alternates between moving the largest unsorted element to its correct position at the end of the array and the smallest unsorted element to its correct position at the beginning.
Example:
def cocktail_shaker_sort(arr):
n = len(arr)
swapped = True
start = 0
end = n-1
while (swapped == True):
# reset the swapped flag on entering the loop,
# because it might be true from a previous
# swap even if there were no swaps made in the
# last iteration.
swapped = False
# loop from left to right same as the bubble sort
for i in range(start, end):
if (arr[i] > arr[i + 1]):
arr[i], arr[i + 1] = arr[i + 1], arr[i]
swapped = True
# if nothing moved, then array is sorted.
if (swapped == False):
break
# otherwise, reset the swapped flag so that it
# can be used in the next stage
swapped = False
# move the end point back by one, because
# item at the end is in its rightful spot
end = end-1
# from right to left, doing the same
# comparison as in the previous stage
for i in range(end-1, start-1, -1):
if (arr[i] > arr[i + 1]):
arr[i], arr[i + 1] = arr[i + 1], arr[i]
swapped = True
# increase the starting point, because
# the last stage would have moved the next
# smallest number to its rightful spot.
start = start + 1
Conclusion
In conclusion, the evolution of bubble sorting has witnessed a remarkable journey from its humble beginnings to the modern implementations we see today. This algorithm, though simple in nature, has inspired numerous enhancements and optimization techniques to overcome its initial limitations. As we marvel at the ingenuity behind bubble sorting variants and its comparison with other sorting algorithms, we realize that even seemingly basic concepts can pave the way for groundbreaking innovations. The evolution of bubble sorting reminds us that progress is not always about reinventing the wheel but rather about refining and optimizing existing solutions, ultimately leading to more efficient and elegant algorithms.