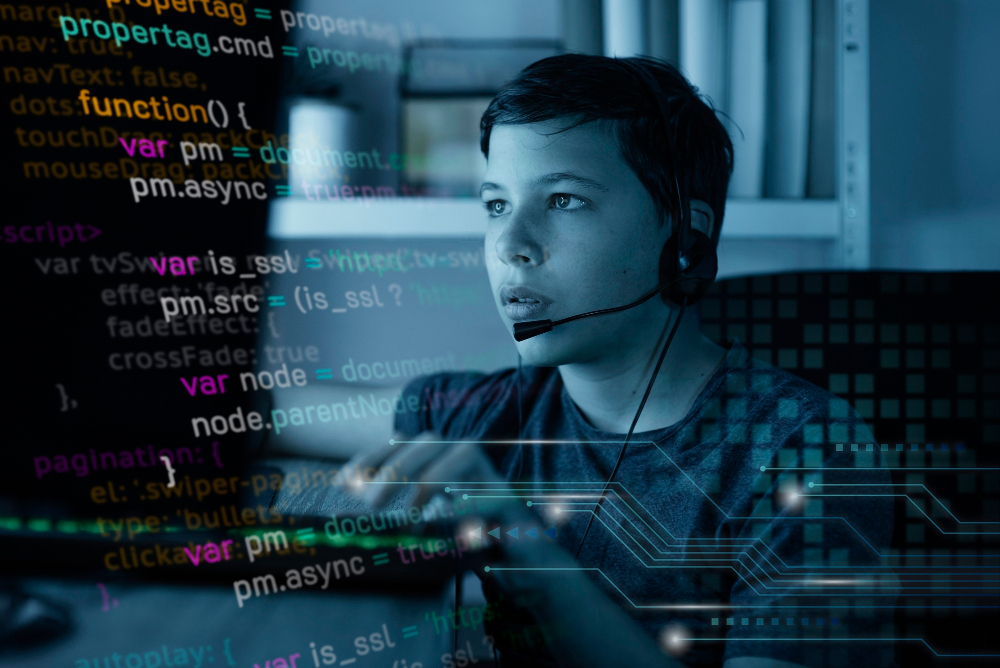
The Power of Operations: Transforming Strings into Equals
In this article, we delve into the powerful realm of operations where we witness the extraordinary transformation of mere strings into equals. Brace yourselves for an insightful journey as we explore the intricate mechanisms that lie behind this fascinating process. Get ready to embark on a captivating quest that will unravel the complexities and unveil the hidden potential of operations. Discover the true significance and impact they can have, as we promise to equip you with the knowledge that will empower your understanding and unlock new possibilities. Get ready to witness the power of operations like never before.
Strings, as sequences of characters, play a pivotal role in computer science and programming. The ability to manipulate and transform strings is crucial for a wide range of applications, from data processing to user interface design. This discussion focuses on the power of operations in transforming strings into equals, exploring the nuanced techniques available in JavaScript.
Understanding String Operations:
Before delving into the examples, it is essential to grasp the fundamental string operations available in JavaScript. These operations include concatenation, substring extraction, replacement, and more. Understanding these operations lays the foundation for the subsequent exploration of transforming strings into equals.
Concatenation:
Concatenation involves combining two or more strings to create a new one. In JavaScript, this can be achieved using the ‘+’ operator. For example:
let str1 = “Hello”;
let str2 = ” World”;
let result = str1 + str2;
console.log(result); // Output: Hello World
Substring Extraction:
Extracting substrings allows developers to isolate specific portions of a string. The `substring()` method in JavaScript is one way to achieve this:
let originalString = “Transforming”;
let extractedString = originalString.substring(0, 5);
console.log(extractedString); // Output: Trans
Replacement:
Replacing segments of a string is another common operation. The `replace()` method can be employed for this purpose:
let sentence = “The quick brown fox jumps over the lazy dog”;
let replacedSentence = sentence.replace(“fox”, “cat”);
console.log(replacedSentence); // Output: The quick brown cat jumps over the lazy dog
The Power of Transformative Operations:
Now, let’s explore how combining these fundamental operations can transform strings into equals, unlocking the potential for creating dynamic and adaptable code.
Example 1: String Reversal
One powerful transformation involves reversing a string. By iterating through the characters and concatenating them in reverse order, we can achieve this effect:
function reverseString(inputString) {
let reversedString = “”;
for (let i = inputString.length – 1; i >= 0; i–) {
reversedString += inputString[i];
}
return reversedString;
}
let original = “Transform”;
let reversed = reverseString(original);
console.log(reversed); // Output: mrofsnarT
Example 2: Anagram Checking
Anagrams are words or phrases formed by rearranging the letters of another. By comparing the sorted versions of two strings, we can determine if they are anagrams:
function areAnagrams(str1, str2) {
let sortedStr1 = str1.split(“”).sort().join(“”);
let sortedStr2 = str2.split(“”).sort().join(“”);
return sortedStr1 === sortedStr2;
}
let word1 = “listen”;
let word2 = “silent”;
console.log(areAnagrams(word1, word2)); // Output: true
Example 3: Palindrome Detection
Detecting palindromes, words or phrases that read the same backward as forward, is another fascinating application of string transformations:
function isPalindrome(inputString) {
let reversedString = inputString.split(“”).reverse().join(“”);
return inputString === reversedString;
}
let phrase = “radar”;
console.log(isPalindrome(phrase)); // Output: true
Advanced Transformations:
Moving beyond basic operations, advanced transformations involve leveraging regular expressions and built-in methods to achieve more complex manipulations.
Example 4: Removing Whitespace
Removing whitespace from a string can enhance data consistency. The `replace()` method coupled with a regular expression can achieve this:
let stringWithWhitespace = ” Trim WhiteSpace “;
let trimmedString = stringWithWhitespace.replace(/\s/g, “”);
console.log(trimmedString); // Output: TrimWhiteSpace
Example 5: Camel Case Conversion
Transforming strings into camel case is often necessary for maintaining a standardized naming convention. The following example demonstrates this transformation:
function toCamelCase(inputString) {
return inputString.replace(/[-_](.)/g, (_, c) => c.toUpperCase());
}
let snake_case = “transforming_strings”;
let camelCase = toCamelCase(snake_case);
console.log(camelCase); // Output: transformingStrings
Understanding the Essence of Operations
Understanding the essence of operations in the context of JavaScript involves grasping the fundamental actions and manipulations that can be performed on data within the language. Operations in JavaScript include various mathematical calculations, logical comparisons, and string manipulations that are essential for programming and web development.
Let’s break down the key aspects:
1. Mathematical Operations:
JavaScript supports standard arithmetic operations like addition (+), subtraction (-), multiplication (*), and division (/). For example:
let result = 5 + 3; // result will be 8
2. Logical Operations:
Logical operations deal with Boolean values (true or false). Examples include AND (`&&`), OR (`||`), and NOT (`!`). They are crucial for decision-making in programming:
let isTrue = true;
let isFalse = false;
let andResult = isTrue && isFalse; // andResult will be false
3. Comparison Operations:
JavaScript allows the comparison of values using operators such as equality (`==` or `===` for strict equality), inequality (`!=` or `!==`), greater than (`>`), less than (`<`), etc. For instance:
let value1 = 10;
let value2 = 5;
let isGreaterThan = value1 > value2; // isGreaterThan will be true
4. String Operations:
Strings in JavaScript can be concatenated using the `+` operator, and various methods are available for string manipulation:
let firstName = “John”;
let lastName = “Doe”;
let fullName = firstName + ” ” + lastName; // fullName will be “John Doe”
5. Increment and Decrement Operations:
JavaScript supports increment (`++`) and decrement (`–`) operators to increase or decrease the value of a variable by 1:
let count = 5;
count++; // count will be 6
Understanding and mastering these operations are fundamental for anyone working with JavaScript, as they form the building blocks for more complex algorithms and functionalities in web development and software engineering. A solid grasp of these concepts is essential for efficient and effective programming in the language.
Exploring the Significance of Strings in Programming
Strings, in the realm of programming, serve as fundamental data types that represent sequences of characters. Understanding their significance is pivotal for any programmer, as they are extensively used for text manipulation, data representation, and various other essential tasks.
In JavaScript, a popular and versatile programming language, strings play a crucial role. They are employed for tasks ranging from basic text output to complex data processing. Let’s delve into a few examples to illustrate their importance:
1. Text Manipulation:
“`javascript
let greeting = “Hello”;
let name = “John”;
let welcomeMessage = greeting + “, ” + name + “!”;
console.log(welcomeMessage);
// Output: Hello, John!
“`
Here, strings are concatenated to create a personalized welcome message.
2. String Methods:
“`javascript
let sentence = “Exploring the significance of strings in programming”;
let wordCount = sentence.split(” “).length;
console.log(“Word count:”, wordCount);
// Output: Word count: 8
“`
The `split` method is used to count the number of words in a sentence, showcasing the versatility of string manipulation in JavaScript.
3. Regular Expressions:
“`javascript
let email = “user@example.com”;
let isValidEmail = /^\S+@\S+\.\S+$/.test(email);
console.log(“Is valid email?”, isValidEmail);
// Output: Is valid email? true
“`
Regular expressions, often applied to strings, can be used for tasks such as email validation, demonstrating the power of strings in handling complex patterns.
4. JSON (JavaScript Object Notation):
“`javascript
let studentInfo = ‘{“name”: “Alice”, “age”: 25, “grade”: “A”}’;
let parsedInfo = JSON.parse(studentInfo);
console.log(“Student Name:”, parsedInfo.name);
// Output: Student Name: Alice
“`
Strings are used to represent and transmit structured data, as exemplified by JSON parsing in JavaScript.
In essence, exploring the significance of strings in programming, particularly in JavaScript, opens up a world of possibilities for developers. Mastery of string manipulation facilitates effective communication, data processing, and the implementation of various algorithms. It is an indispensable skill for any programmer aiming to harness the full potential of the programming language.
Conclusion
The transformative capabilities of operations when applied to strings are truly awe-inspiring. From performing arithmetic operations to comparison and logical operations, strings can be molded and reshaped into equals, unlocking a multitude of possibilities in programming. As we delve deeper into the world of string transformations, it becomes evident that with the right combination of operations, we can create elegant solutions to complex problems. So, embrace the power of operations and let your imagination soar as you harness the potential of strings in your programming endeavors—where there were once disparate elements, you now have the tools to unite them into equals.