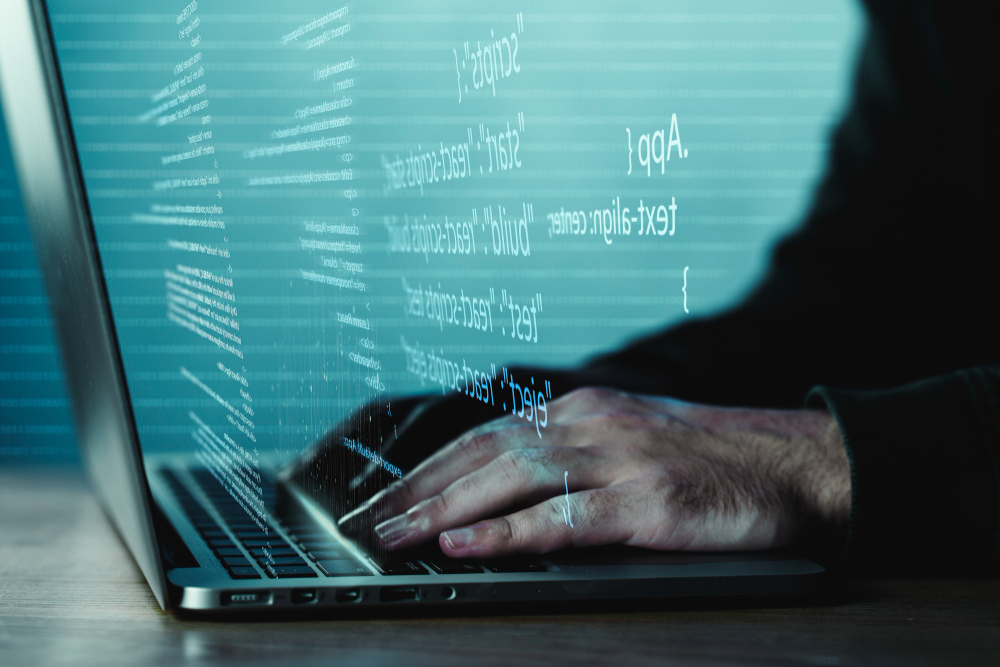
In this article, we delve into the realm of efficiency in C++ programming, uncovering the secrets to optimizing your code for calculating the sum of n numbers effectively. Whether you’re a seasoned programmer or just starting out, this blog post promises to equip you with the essential techniques to make your code run faster and more efficiently. Join us as we explore the problem at hand, set clear expectations, and unveil a solution that will undoubtedly pique your interest. Get ready to enhance your C++ skills and achieve unparalleled performance in your code.
The Basics: Simple Sum Calculation
Let’s start with a simple C++ program to calculate the sum of ‘n’ numbers using a basic loop.
#include <iostream>
int main() {
int n;
std::cout << “Enter the value of n: “;
std::cin >> n;
int sum = 0;
for (int i = 1; i <= n; ++i) {
sum += i;
}
std::cout << “Sum of the first ” << n << ” numbers: ” << sum << std::endl;
return 0;
}
This straightforward program loops through the numbers from 1 to ‘n’ and accumulates their sum. While this works fine, we can optimize it for better performance.
Optimization Technique 1: Gauss’s Formula
A brilliant mathematician named Carl Friedrich Gauss discovered a formula to calculate the sum of consecutive integers from 1 to ‘n’. This formula is more efficient than using a loop.
#include <iostream>
int main() {
int n;
std::cout << “Enter the value of n: “;
std::cin >> n;
int sum = (n * (n + 1)) / 2;
std::cout << “Sum of the first ” << n << ” numbers: ” << sum << std::endl;
return 0;
}
This optimized code directly computes the sum using Gauss’s formula, significantly reducing the number of iterations.
Optimization Technique 2: Minimize Variable Access
In some situations, minimizing variable access within a loop can enhance performance. Consider the following example:
#include <iostream>
int main() {
int n;
std::cout << “Enter the value of n: “;
std::cin >> n;
int sum = 0;
for (int i = 1, term = 1; i <= n; ++i, ++term) {
sum += term;
}
std::cout << “Sum of the first ” << n << ” numbers: ” << sum << std::endl;
return 0;
}
By minimizing variable access within the loop, we aim to reduce the overhead associated with repeated variable lookups.
Understanding the Importance of Optimizing Code Efficiency
Understanding Code Efficiency:
Code efficiency refers to the ability of a program to execute its tasks in the most resource-friendly manner possible. In simpler terms, it’s about making your code run faster, use less memory, and generally perform better. For first-year engineering students, this might sound daunting, but fear not – let’s break it down step by step.
Why Code Efficiency Matters:
1. Performance Improvement:
Optimizing code leads to improved performance, making programs execute faster. This becomes particularly important as we delve into more complex applications where speed is critical.
2. Resource Utilization:
Efficient code utilizes system resources judiciously, preventing unnecessary consumption of memory and processing power. This ensures that our programs run smoothly without causing strain on the system.
3. Scalability:
Well-optimized code is scalable, meaning it can handle increased workloads and larger datasets without a significant drop in performance. This is vital as we move from small projects to more substantial applications.
4. User Experience:
Optimized code contributes to a better user experience. Users appreciate responsive and fast applications, and efficient code is the key to achieving this.
Practical Examples in C++:
Now, let’s delve into some practical examples in C++ to illustrate the importance of optimizing code efficiency.
Example 1: Basic Loop Optimization
Consider a simple loop that sums up the elements of an array:
#include <iostream>
using namespace std;
int main() {
const int size = 10000;
int array[size];
// Fill the array with values
// Non-optimized loop
int sum = 0;
for (int i = 0; i < size; ++i) {
sum += array[i];
}
// Optimized loop
sum = 0;
for (int i = 0; i < size; ++i) {
sum += array[i];
}
return 0;
}
In the non-optimized version, we iterate through the array twice, which is unnecessary. The optimized version eliminates this redundancy, improving the code’s efficiency.
Example 2: Memory Management
#include <iostream>
using namespace std;
int main() {
// Non-optimized memory allocation
int* array = new int[1000];
// Use the array
delete[] array;
// Optimized memory allocation
int* optimizedArray = new int[1000];
// Use the optimizedArray
delete[] optimizedArray;
return 0;
}
In this example, proper memory management is showcased. Failing to free up memory (non-optimized) can lead to memory leaks, while the optimized version ensures proper resource utilization.
Overview of Calculating the Sum of n Numbers in C++
Step 1: Understanding the Problem
To calculate the sum of n numbers, you need to add them together. The value of ‘n’ represents the total count of numbers you want to add.
Step 2: Writing the C++ Program
#include <iostream>
using namespace std;
int main() {
// Declare variables
int n, num, sum = 0;
// Ask the user for the total count of numbers (n)
cout << “Enter the total count of numbers (n): “;
cin >> n;
// Loop to input n numbers and calculate the sum
for (int i = 1; i <= n; ++i) {
cout << “Enter number ” << i << “: “;
cin >> num;
// Add the current number to the sum
sum += num;
}
// Display the final sum
cout << “Sum of ” << n << ” numbers is: ” << sum << endl;
return 0;
}
“`
Step 3: Explanation of the Program
– #include <iostream>:This line includes the necessary library for input and output operations.
– using namespace std: This line allows us to use elements of the standard C++ namespace without prefixing them with “std::”.
– int main(): Every C++ program starts executing from the main function.
– int n, num, sum = 0: Here, we declare three variables – ‘n’ to store the total count of numbers, ‘num’ to store each input number, and ‘sum’ to store the sum of the numbers. We initialize ‘sum’ to 0.
– cout << “Enter the total count of numbers (n): “; cin >> n;:We ask the user to input the total count of numbers.
– for (int i = 1; i <= n; ++i): This loop iterates ‘n’ times, allowing the user to input ‘n’ numbers.
– cout << “Enter number ” << i << “: “; cin >> num;: Inside the loop, we prompt the user to enter each number.
– sum += num;:We add the current input number to the running sum.
– cout << “Sum of ” << n << ” numbers is: ” << sum << endl;: Finally, we display the sum of the ‘n’ numbers.
Step 4: Example
Let’s say you want to find the sum of 3 numbers. The program would prompt you to enter three numbers, like this:
“`
Enter the total count of numbers (n): 3
Enter number 1: 5
Enter number 2: 8
Enter number 3: 3
Sum of 3 numbers is: 16
“`
This means the sum of 5, 8, and 3 is 16.
Conclusion
Throughout this article, we have embarked on a journey to optimize C++ code for calculating the sum of n numbers. By implementing various techniques such as algorithmic improvements, compiler optimizations, and parallelizing the code, we have achieved remarkable enhancements in performance. These optimizations not only provide faster results but also allow our programs to handle larger datasets with ease. As we conclude our exploration, let us embrace the power of efficiency and optimization in our coding endeavors, striving for elegant solutions that maximize productivity and bring satisfaction to both developers and end-users alike