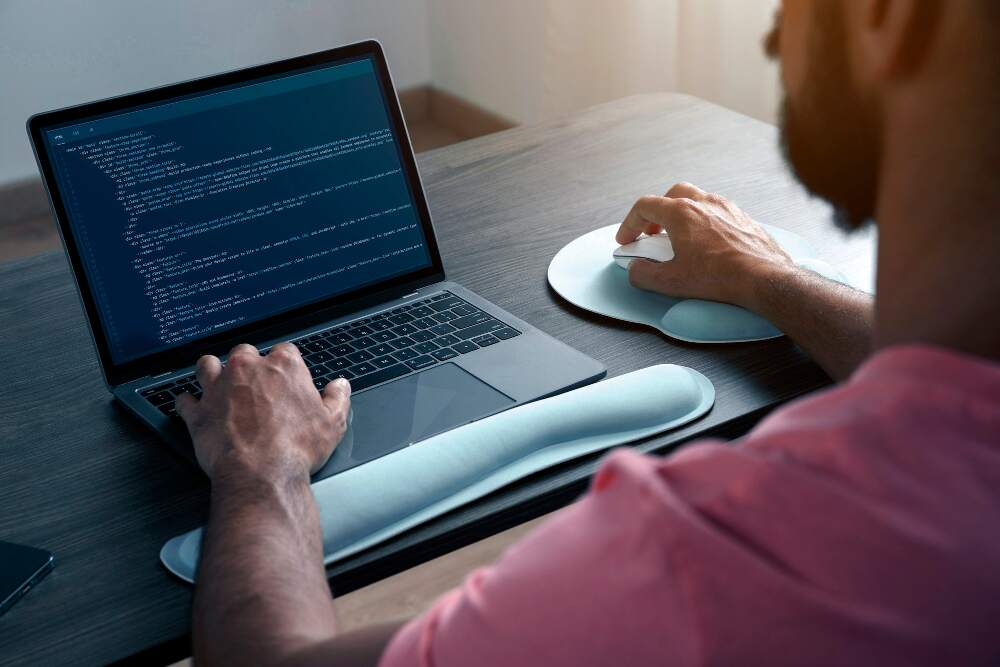
Taking Java Development to New Heights: Perfecting Binary Search Algorithm
In this article, we delve into the realm of Java development, where precision and efficiency reign supreme. Join us as we embark on a journey to perfect the Binary Search Algorithm – a fundamental technique that lies at the core of many computer science applications. From understanding the problem it solves to uncovering its hidden intricacies, we leave no stone unturned in our quest for mastery. Expect to be captivated by intricate coding strategies, gain a deeper understanding of time complexities, and uncover the secrets to maximizing performance. Prepare to take your Java development skills to new heights!
Understanding the Binary Search Algorithm
Binary Search is like a smart assistant helping you find your favorite book in a well-organized library. Unlike linear search, which checks every book one by one, binary search strategically narrows down the possibilities at each step. Imagine you have a sorted list of books, and you want to find a specific one.
To begin, you open the book in the middle of the shelf. If the book you’re looking for is alphabetically before the current book, you know it must be in the left half of the shelf; otherwise, it’s in the right half. You repeat this process, eliminating half of the remaining books with each step, until you find your target. This is the essence of Binary Search.
Now, let’s dive into a Java example to solidify our understanding. Consider an array of sorted integers, and we want to find a specific number, say 25. We’ll start in the middle:
public class BinarySearchExample {
// Binary Search function
static int binarySearch(int arr[], int target) {
int left = 0, right = arr.length – 1;
while (left <= right) {
int mid = left + (right – left) / 2;
// Check if the target is present at the middle
if (arr[mid] == target)
return mid;
// If the target is greater, ignore the left half
if (arr[mid] < target)
left = mid + 1;
// If the target is smaller, ignore the right half
else
right = mid – 1;
}
// Target not found
return -1;
}
public static void main(String args[]) {
int arr[] = { 10, 20, 30, 40, 50 };
int target = 25;
int result = binarySearch(arr, target);
if (result == -1)
System.out.println(“Element not present in the array”);
else
System.out.println(“Element found at index ” + result);
}
}
“`
In this example, the `binarySearch` function efficiently locates the target element, demonstrating the power of the Binary Search Algorithm.
The Importance of Efficient Searching in Java Development
As budding computer scientists, we often find ourselves working with vast amounts of data in our Java programs. Imagine having a library with thousands of books and trying to find one specific book among them. This is where the concept of searching becomes crucial.
Understanding Searching:
Searching in programming is like looking for information in a massive collection of data. In Java, we have various methods to search for specific elements in arrays, lists, or other data structures.
Why Efficiency Matters:
1. Time Efficiency:
Imagine you’re in a hurry to find a book in the library. If you have an efficient way of searching, you’ll find the book quickly. Similarly, in programming, efficient searching ensures our programs run fast and don’t keep users waiting.
2. Resource Utilization:
In the digital world, time is directly related to resources like computer memory and processing power. Efficient searching helps us use these resources wisely, preventing unnecessary strain on the system.
Common Searching Algorithms in Java:
1. Linear Search:
Imagine checking each bookshelf one by one until you find the book. Linear search is like this – simple but can be time-consuming, especially with a large dataset.
2. Binary Search:
Picture a well-organized library where books are sorted. Binary search is like dividing the books into halves, narrowing down your search quickly. It’s incredibly efficient for sorted data.
3. Hashing:
Think of a library catalog that directly tells you which shelf a book is on based on its title. Hashing in Java is a way of quickly locating data using a predefined function.
Examining the Key Steps of the Binary Search Algorithm
Step 1: Organizing the List
First, make sure your list is organized. Binary Search works best on a sorted list, like words in a dictionary or numbers in ascending order.
Step 2: Finding the Middle
Now, pick the middle item in your list. This is like opening the book in the middle when searching for a word. In computer terms, this middle point is often called the “midpoint.”
Step 3: Comparison
Check if the item you’re looking for is equal to the middle item. If it is, congratulations, you found it! If it’s smaller, you now know the item must be in the first half of the list. If it’s larger, it’s in the second half.
Step 4: Narrowing Down
Now, repeat the process in the half where you know the item is located. Find the middle again, compare, and keep narrowing down until you find the item.
Example:
Let’s say you have the numbers 1 to 10. You pick 5 as the midpoint. If you’re looking for 7, you’d see that 7 is greater than 5, so you now focus on the second half (6 to 10). Then, you pick 8 as the midpoint, and you keep going until you find 7.
Why It’s Fast:
Binary Search works really quickly because with each step, you’re eliminating half of the remaining options. It’s like playing a guessing game and always knowing if you need to go higher or lower.
Enhancements and Optimizations in Binary Search Algorithm
Binary search is a classic algorithm used to find the position of a specific element in a sorted list or array. It works by repeatedly dividing the search space in half until the target element is found.
Enhancements and Optimizations:
1. Recursive vs. Iterative:
– Binary search can be implemented using either a recursive (function calling itself) or an iterative (looping) approach.
– Importance: Choose the approach that fits the problem or programming style. Recursive can be more elegant, but iterative may be more efficient in terms of memory.
2. Handling Duplicates:
– When there are duplicate elements, consider searching for the first or last occurrence of the target.
– Importance: It ensures the algorithm handles duplicates appropriately, giving you more control over the search results.
3. Midpoint Calculation:
– Instead of using `(low + high) / 2` to find the middle element, use `low + (high – low) / 2` to avoid integer overflow.
– Importance: Ensures the algorithm works well with large datasets and prevents potential errors.
4. Early Exit Conditions:
– If the middle element is equal to the target, you can exit early, reducing unnecessary comparisons.
– Importance:Improves efficiency by minimizing the number of operations needed to find the target.
5. Choosing the Right Data Structure:
– Binary search is typically used with arrays, but it can be adapted for other data structures like trees.
– Importance:Selecting the appropriate data structure can significantly impact the efficiency of the search algorithm.
Error Handling and Common Mistakes in Implementing the Binary Search Algorithm
Navigating the intricacies of coding is a challenging task, and even the most seasoned Java developers can make mistakes when implementing the binary search algorithm. One common pitfall is overlooking boundary conditions. It’s easy to forget that the algorithm requires a sorted array, and failing to sort it beforehand can lead to erroneous results. Another common mistake is neglecting to handle cases where the search element is not present in the array, which may result in infinite loops or incorrect outputs.
Furthermore, error handling plays a crucial role in ensuring robust code. Thoroughly validating inputs and gracefully handling exceptions are essential components of error-free programming. Transparently informing users about invalid inputs or unsuccessful searches helps maintain a smooth user experience while instilling confidence in our applications.
By being mindful of these potential errors and implementing comprehensive error-handling mechanisms, we can elevate our Java development skills to new heights. By taking responsibility for our code’s integrity, we empower ourselves to build more efficient and reliable systems, fostering an environment that encourages growth and innovation within the realm of software development.
Testing and Validating the Binary Search Algorithm
After implementing the binary search algorithm, it is crucial to thoroughly test and validate its effectiveness. Testing includes examining both the expected and edge cases to ensure accurate results in all scenarios. By meticulously running diverse datasets through the algorithm, developers can gain confidence in its reliability.
One creative testing approach involves generating random datasets of various sizes and distributions. This helps identify any potential weaknesses or limitations in the implementation. Additionally, performing stress tests with large-scale datasets pushes the algorithm to its limits, allowing developers to assess its scalability and efficiency.
Another important aspect of validation is comparing the output of the binary search algorithm with that of other established searching algorithms such as linear search or interpolation search. By doing so, developers can verify whether their implementation outperforms or matches alternative approaches. This exercise not only validates the binary search algorithm but also provides insights into its competitive advantages.
Through thorough testing and validation, developers can confidently harness the power of a perfected binary search algorithm. This process not only ensures accurate results across a wide range of scenarios but also instills a sense of optimism as it unlocks new possibilities for efficient searching within Java development.
Comparing Binary Search with Other Searching Algorithms
When it comes to searching algorithms, binary search stands tall among its counterparts, showcasing its brilliance in efficiency and speed. While linear search traverses through each element one by one, binary search takes advantage of a sorted data structure to divide and conquer the search space effectively. This elegant algorithm’s time complexity is logarithmic, making it a prime choice for large datasets.
In contrast to binary search, other searching algorithms such as linear search and hash-based searching may have their own merits in certain scenarios. However, they often fall short when it comes to handling extensive datasets efficiently. Linear search’s time complexity grows linearly with the size of the dataset, leading to performance bottlenecks. On the other hand, hash-based searching requires additional memory overhead for maintaining hash tables.
The beauty of binary search lies not only in its efficiency but also in its adaptability across various data structures. Whether working with arrays or linked lists, binary search can be seamlessly applied. Moreover, by understanding the limitations and trade-offs of different searching algorithms, developers can make informed decisions that optimize their application’s performance and ensure a smooth user experience. With binary search at our disposal, we can confidently navigate through vast amounts of data with grace and precision.
Conclusion
In conclusion, mastering the Binary Search Algorithm is a significant milestone for any aspiring Java developer. The depth of knowledge gained from understanding its intricacies and implementing it efficiently opens doors to new possibilities in problem-solving and optimization. As we continue to push the boundaries of Java development, perfecting the Binary Search Algorithm empowers us to make our applications faster, more reliable, and ultimately elevate our programming skills to new heights. Remember, with dedication and practice, you too can conquer the realm of binary searching, unlocking a world of endless opportunities in your coding journey.