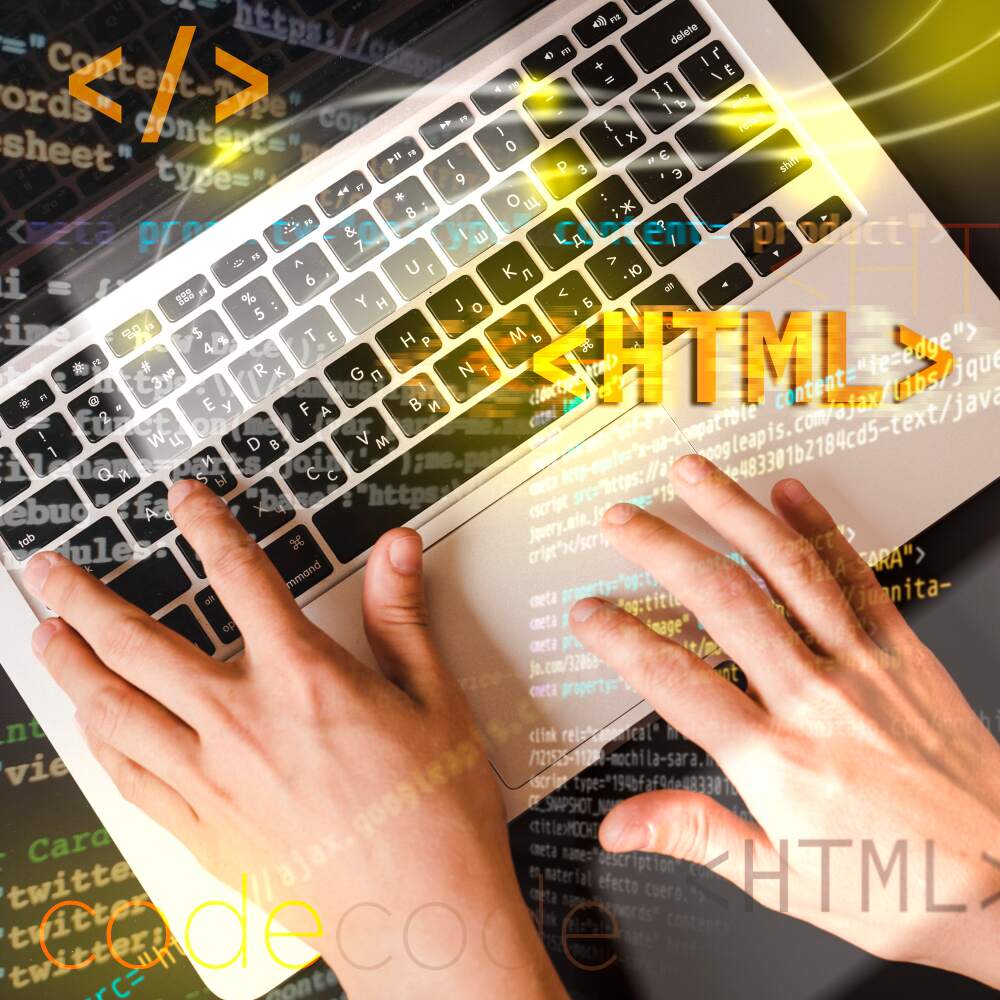
Introduction:
Greetings, aspiring engineers! As a professor with over a decade of experience in the field of Computer Science, I understand the importance of mastering fundamental data structures. Today, let’s delve into the world of linked lists, a crucial concept that forms the backbone of many algorithms and applications.
Linked lists are dynamic data structures that allow for efficient storage and manipulation of data. Unlike arrays, linked lists provide flexibility in terms of size and memory allocation, making them a key component in the arsenal of any skilled programmer. In this blog, we’ll explore the implementation of linked lists in Python, breaking down the complexities to ensure that even beginners can grasp the concepts.
Understanding Linked Lists:
At its core, a linked list is a collection of nodes, where each node holds a data element and a reference (or link) to the next node in the sequence. This dynamic structure enables us to insert, delete, and traverse elements with ease.
Let’s start by implementing a simple singly linked list in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def display(self):
current = self.head
while current:
print(current.data, end=” -> “)
current = current.next
print(“None”)
In this example, the Node class represents each element in the linked list, while the LinkedList class provides methods for appending data and displaying the list.
Usage Example:
# Creating a linked list
my_linked_list = LinkedList()
# Appending elements
my_linked_list.append(10)
my_linked_list.append(20)
my_linked_list.append(30)
# Displaying the linked list
my_linked_list.display()
The output will be: 10 -> 20 -> 30 -> None
Conclusion:
In conclusion, understanding linked lists is a crucial step in your journey as a programmer. The implementation in Python presented here is just the tip of the iceberg. As you continue your exploration, you’ll encounter doubly linked lists, circular linked lists, and various optimization techniques.
Mastery of linked lists not only enhances your problem-solving skills but also lays a solid foundation for more complex data structures and algorithms. As engineering students, embracing these fundamental concepts will empower you to tackle real-world challenges with confidence. Happy coding!