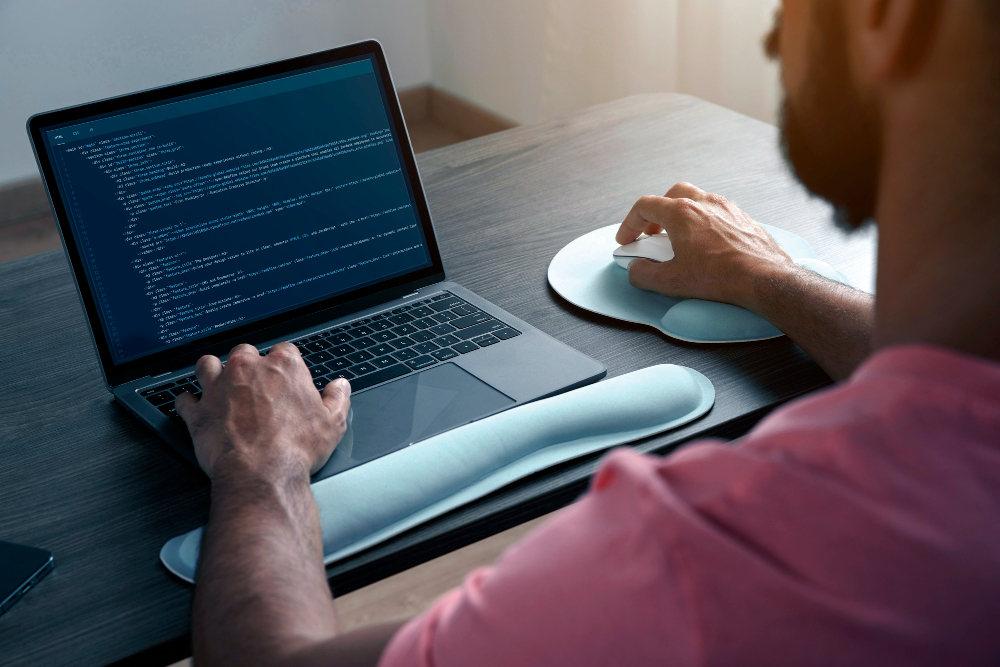
Introduction:
Linked lists are fundamental data structures used in computer science and programming. They provide a dynamic way of organizing and storing data, offering flexibility in memory management. As a lecturer with over 10 years of experience, let’s delve into the intricacies of implementing linked lists in Python, exploring their structure, operations, and practical applications.
A linked list consists of nodes, each containing data and a reference to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory allocation, allowing for efficient insertion and deletion of elements. Understanding the implementation of linked lists is crucial for aspiring programmers and computer science enthusiasts.
Basic Structure of a Linked List:
A linked list comprises nodes, and the first node is called the head. Each node contains data and a reference (or link) to the next node in the sequence. The last node points to null, indicating the end of the list. This structure allows for easy traversal and manipulation of data.
Types of Linked Lists:
1. Singly Linked List: Each node points to the next node in the sequence, forming a unidirectional chain.
2. Doubly Linked List: Nodes have references to both the next and previous nodes, enabling bidirectional traversal.
3. Circular Linked List: The last node points back to the first, forming a closed loop.
Implementing a Singly Linked List in Python:
Let’s explore a basic implementation of a singly linked list in Python, covering key operations such as insertion, deletion, and traversal.
“`python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def insert_at_end(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def delete_node(self, key):
current = self.head
if current and current.data == key:
self.head = current.next
current = None
return
prev = None
while current and current.data != key:
prev = current
current = current.next
if current is None:
return
prev.next = current.next
current = None
def display(self):
current = self.head
while current:
print(current.data, end=” -> “)
current = current.next
print(“None”)
“`
Example Usage:
“`python
# Creating a linked list
linked_list = LinkedList()
linked_list.insert_at_end(10)
linked_list.insert_at_end(20)
linked_list.insert_at_end(30)
linked_list.display() # Output: 10 -> 20 -> 30 -> None
# Deleting a node
linked_list.delete_node(20)
linked_list.display() # Output: 10 -> 30 -> None
“`
Conclusion:
Understanding linked lists is foundational in programming, as they provide a dynamic and efficient way to manage data. In this guide, we’ve explored the basic structure of linked lists and implemented a simple singly linked list in Python, covering insertion, deletion, and traversal operations. As educators and learners, grasping these concepts lays the groundwork for tackling more complex data structures and algorithms, contributing to a robust skill set in the world of computer science and programming.