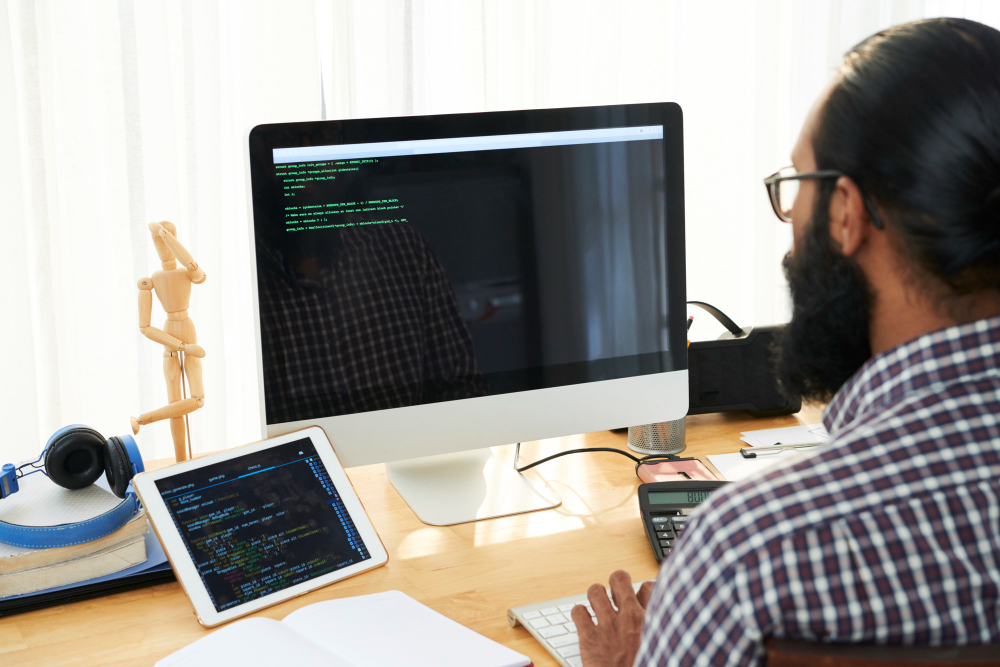
Welcome, future innovators! Python is a fantastic language to learn. Its clear syntax and vast libraries make it perfect for tackling various problems, from data analysis to building websites.
This blog dives into some simple Python problems designed to solidify your understanding of core concepts like variables, data types, loops, and conditional statements. By working through these problems and their solutions, you’ll gain valuable experience in applying Python’s power to solve practical tasks.
Getting Started:
Before we begin, ensure you have Python installed on your computer. You can download it for free from Python. Once installed, you can launch the Python interpreter by typing “python” in your terminal or command prompt.
Problem 1: Area and Perimeter of a Rectangle
Let’s start with a classic! We want to calculate the area and perimeter of a rectangle given its length and width.
Solution:
# Define variables for length and width
length = float(input(“Enter the length of the rectangle: “))
width = float(input(“Enter the width of the rectangle: “))
# Calculate area and perimeter
area = length * width
perimeter = 2 * (length + width)
# Print the results
print(“Area of the rectangle:”, area)
print(“Perimeter of the rectangle:”, perimeter)
Explanation:
- We use the input function to take user input for the length and width (converted to floats for decimal values).
- The area is calculated by multiplying length and width.
- The perimeter is calculated by adding twice the length and width.
- Finally, we use print statements to display the calculated area and perimeter.
Problem 2: Check if a Number is Even or Odd
This problem tests your grasp of conditional statements. We want to determine if a given number is even or odd.
Solution:
# Get a number from the user
number = int(input(“Enter a number: “))
# Check if the number is even using modulo operator
if number % 2 == 0:
print(“The number is even”)
else:
print(“The number is odd”)
Explanation:
- We use input to get a number and convert it to an integer using int.
- The modulo operator (%) gives the remainder after division. Here, we check if the remainder is 0.
- If the remainder is 0, the number is even (divisible by 2). Otherwise, it’s odd.
Problem 3: Find the Largest Number
Let’s explore loops with a problem where we find the largest number among three user inputs.
Solution:
# Initialize a variable to store the largest number
largest = None
# Get three numbers from the user
for i in range(3):
number = int(input(“Enter number ” + str(i+1) + “: “))
if largest is None or number > largest:
largest = number
# Print the largest number
print(“The largest number is:”, largest)
Explanation:
- We initialize a variable largest to hold the biggest number we encounter.
- We use a for loop to iterate three times (range(3)).
- Inside the loop, we get a number from the user and convert it to an integer.
- We use an if statement to check if largest is empty or the current number is bigger than the current largest.
- If the condition is true, we update largest with the current number.
- After the loop finishes, largest holds the biggest number entered.
Problem 4: Calculate the Simple Interest
This problem applies formulas and variables, simulating a real-world financial calculation. We want to find the simple interest for a given principal amount, time period, and interest rate.
Solution:
# Get principal amount, time, and interest rate from the user
principal = float(input(“Enter the principal amount: “))
time = float(input(“Enter the time period (in years): “))
rate = float(input(“Enter the interest rate (in %): “))
# Convert interest rate to decimal
rate = rate / 100
# Calculate simple interest
simple_interest = principal * time * rate
# Print the simple interest
print(“The simple interest is:”, simple_interest)
Explanation:
- We get user input for principal amount, time, and interest rate, converting them to appropriate data types.
- We convert the interest rate