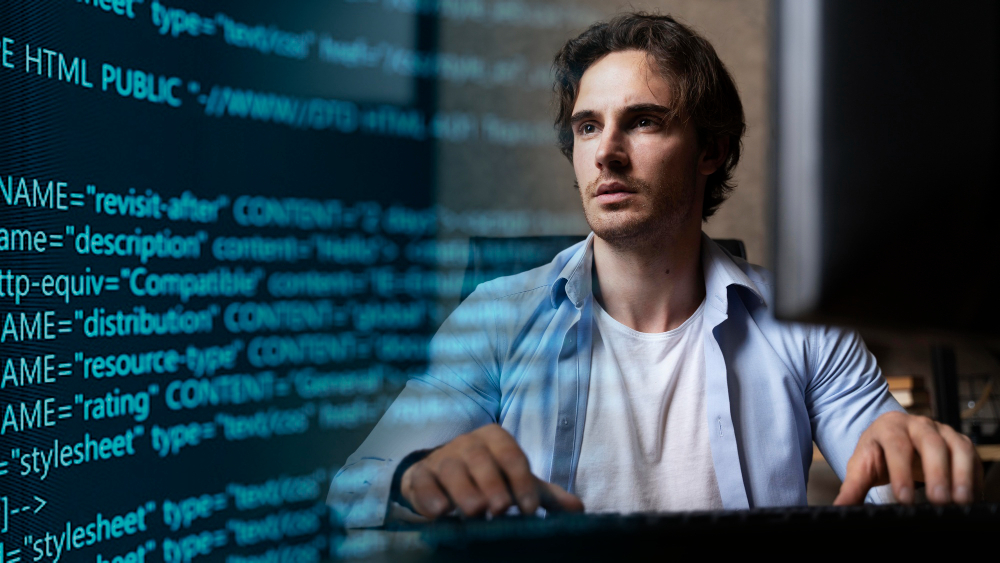
Introduction
Programming math problems aren’t just about memorizing formulas (although some basic understanding is helpful). They’re about training your brain to think logically, solve problems efficiently, and translate real-world scenarios into code. Here’s why they’re important and some fun examples to get you started.
Why are Programming Math Problems Important?
Think of programming as building a house. You need a blueprint (your code), strong materials (algorithms and data structures), and a solid foundation (your mathematical understanding). Programming math problems help you build that foundation by:
- Developing problem-solving skills: These problems often involve real-world situations like calculating distances, optimizing resource allocation, or analyzing data sets. You’ll learn to break down problems into smaller, solvable steps – a crucial skill for any programmer.
- Understanding algorithms: Many programming algorithms rely on mathematical concepts. For example, sorting algorithms like quicksort utilize concepts like recursion and partitioning. By mastering these math concepts, you’ll grasp algorithms at a deeper level.
- Optimizing code: Programming is all about efficiency. Math problems help you understand things like time and space complexity, allowing you to write cleaner, more efficient code that runs faster and uses less memory.
Level Up Your Skills with Fun Math Problems
Here are some engaging programming math problems to test your skills and make learning fun. Feel free to use any programming language you’re comfortable with:
Problem 1: Area and Perimeter Calculator
This is a classic, but a great way to start. Write a program that takes the length and width of a rectangle as input and calculates its area and perimeter. This involves basic arithmetic operations:
length = float(input(“Enter the length: “))
width = float(input(“Enter the width: “))
area = length * width
perimeter = 2 * (length + width)
print(“Area:”, area)
print(“Perimeter:”, perimeter)
Problem 2: Distance Calculator
Level up by incorporating the Pythagorean theorem! Write a program that takes the coordinates (x1, y1) and (x2, y2) of two points as input and calculates the distance between them. Remember the formula:
x1 = float(input(“Enter x-coordinate of point 1: “))
y1 = float(input(“Enter y-coordinate of point 1: “))
x2 = float(input(“Enter x-coordinate of point 2: “))
y2 = float(input(“Enter y-coordinate of point 2: “))
distance = ((x2 – x1) ** 2 + (y2 – y1) ** 2) ** 0.5
print(“Distance between points:”, distance)
Problem 3: Prime Number Checker
Dive into loops and conditional statements by writing a program that checks if a given number is prime. A prime number is only divisible by 1 and itself. Here’s the logic:
number = int(input(“Enter a number: “))
isPrime = True
if number <= 1:
isPrime = False
else:
for i in range(2, number):
if number % i == 0:
isPrime = False
break
if isPrime:
print(number, “is a prime number.”)
else:
print(number, “is not a prime number.”)
Challenge Yourself:
- Modify the Area and Perimeter Calculator to work with different shapes like circles and triangles.
- In the Distance Calculator, incorporate functions to calculate the distance between different geometric shapes (e.g., point and line).
- For the Prime Number Checker, try finding all prime numbers within a given range.
These are just a few examples, and the possibilities are endless! Don’t be afraid to experiment and explore different types of problems. There are tons of online resources and coding platforms that offer practice problems with varying difficulty levels.
Conclusion
By embracing programming math problems, you’ll not only strengthen your mathematical foundation but also become a better problem solver and a more efficient coder. The journey to becoming a coding master is a continuous learning process. So, buckle up, have fun with these problems, and get ready to impress recruiters with your sharp programming skills.