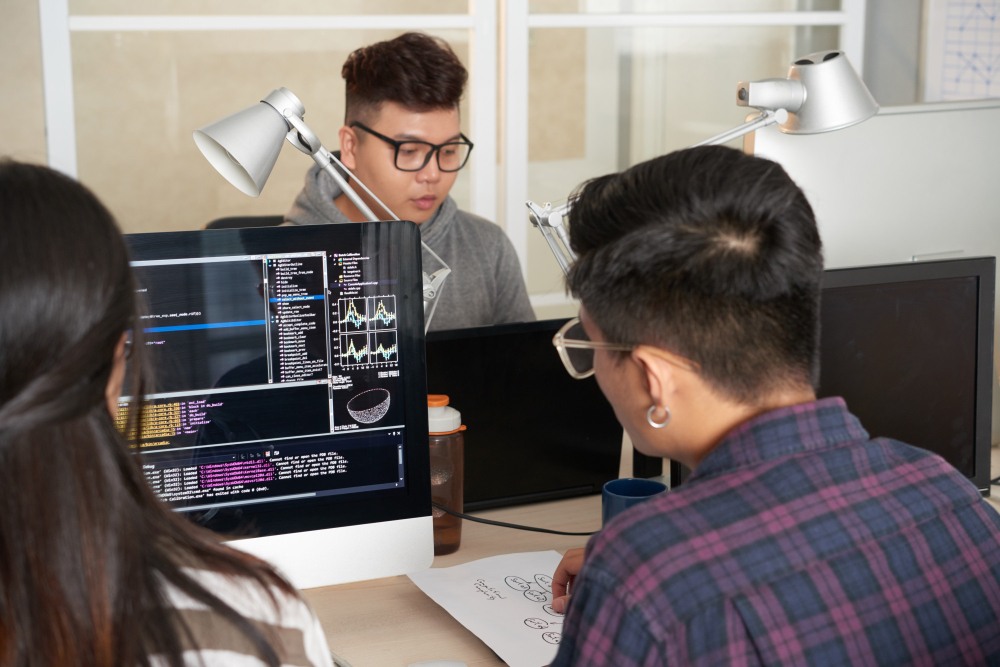
Hey there, future software engineers! Today, we’re diving into the world of technical interviews, specifically a fictional challenge named “T2 Coding Challenge 3.” While this might not be a real interview question, it serves as a great example to prepare you for the types of problems you might encounter during your first year of engineering!
Welcome to the Exciting World of Coding Challenges!
Technical interviews often involve coding challenges to assess your problem-solving skills and coding proficiency. These challenges typically involve writing code to solve a specific task. Don’t worry; they’re not designed to be impossible! They’re meant to gauge your understanding of programming fundamentals and your ability to approach problems logically.
Understanding T2 Coding Challenge 3
Now, let’s dissect our imaginary “T2 Coding Challenge 3.” The specific details will depend on the company and role, but here’s a general idea of what it might entail:
- The Task: Imagine you’re building a music player app. The challenge might ask you to write code that calculates the total duration (in minutes) of a playlist created by the user.
- The Input: The input could be an array containing the durations (in minutes) of individual songs in the playlist.
- The Output: Your code should return the total duration of the playlist by summing the durations of all the songs in the array.
Breaking Down the Problem
Before you start coding, take a moment to understand the problem. Here’s how to approach it:
- Identify Inputs and Outputs: Clearly define what the code receives (the array of song durations) and what it needs to produce (the total playlist duration).
- Plan Your Approach: Think about how you would solve this problem manually. You’d likely loop through the array, adding the duration of each song to a running total. Translate this logic into code.
Coding the Solution (Example in Python):
Here’s an example solution in Python that tackles the T2 Coding Challenge 3:
def calculate_playlist_duration(song_durations):
“””
This function calculates the total duration of a playlist.
Args:
song_durations: A list containing the durations (in minutes) of individual songs.
Returns:
The total duration of the playlist (in minutes).
“””
total_duration = 0
for song_duration in song_durations:
total_duration += song_duration
return total_duration
# Example usage
songs = [3, 5, 4] # Playlist with three songs
total_time = calculate_playlist_duration(songs)
print(f”Total playlist duration: {total_time} minutes”)
Explanation:
- We define a function calculate_playlist_duration that takes an array song_durations as input.
- We initialize a variable total_duration to 0, which will store the accumulated song duration.
- We use a for loop to iterate through each song duration in the song_durations array.
- Inside the loop, we add the current song’s duration to the total_duration variable.
- After the loop, the total_duration variable holds the sum of all song durations.
- The function returns the total_duration.
Testing Your Code:
Always test your code with different inputs to ensure it works as expected. In this case, you can create sample playlists with varying numbers of songs and durations.
Beyond T2 Coding Challenge 3
This example serves as a stepping stone. As you progress, coding challenges will become more complex. Here are some tips for future success:
- Practice, Practice, Practice: The more you code, the better you’ll become at problem-solving and translating logic into code.
- Learn Data Structures and Algorithms: Understanding fundamental data structures (like arrays) and algorithms (like looping) will equip you to tackle diverse coding problems.
- Don’t Be Afraid to Ask Questions: During interviews, clarify any doubts you have about the challenge. Asking questions shows your eagerness to learn and understand the problem.
- Embrace Online Resources: There are tons of online resources with coding challenges and tutorials! Utilize them to enhance your skills.
Conclusion
Coding challenges are an opportunity to showcase your problem-solving skills and passion for coding. By understanding the core concepts and practicing regularly, you’ll be well-equipped to conquer any coding challenge thrown your way during your job search. Keep learning, keep practicing, and best of luck on your coding journey!