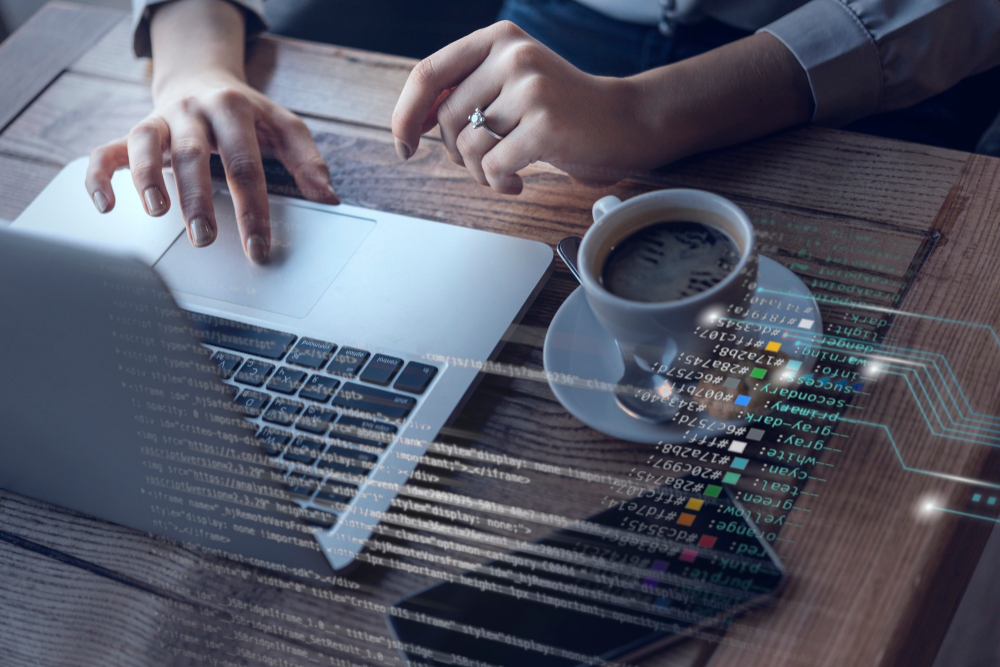
Introduction
Sorting is a fundamental operation in computer science, and understanding efficient sorting algorithms is crucial for writing faster and more effective programs. In this session, we will explore the Merge Sort algorithm, a powerful and efficient way to sort data. We’ll dive into the details with a practical example in C++.
Merge Sort is a divide-and-conquer algorithm. It works by dividing the input array into two halves, sorting each half separately, and then merging the sorted halves. This process continues recursively until the entire array is sorted.
Understanding Sorting Algorithms
Why Sorting Matters:
Imagine you have a list of numbers, and you want to arrange them in ascending or descending order. This process is called sorting, and it’s essential in various applications, such as searching, data analysis, and more. We’ll explore how Merge Sort can help us achieve this task with efficiency.
Merge Sort Basics:
Merge Sort is a divide-and-conquer algorithm, which means it breaks the problem into smaller sub-problems and solves them independently. Here’s a simple analogy: imagine sorting a deck of cards. Divide the deck into two halves, sort each half individually, and then merge them back together in a sorted manner.
Step-by-Step Example:
1. Divide:
– Take an unsorted list of numbers: [4, 2, 7, 1, 5, 3, 6].
– Divide the list into two halves: [4, 2, 7] and [1, 5, 3, 6].
2. Sort:
– Sort each half independently:
– [4, 2, 7] becomes [2, 4, 7].
– [1, 5, 3, 6] becomes [1, 3, 5, 6].
3. Merge:
– Merge the two sorted halves back together:
– Compare the first elements of each half (2 and 1), choose the smaller one (1), and put it in the new list.
– Move to the next elements, compare (2 and 3), choose the smaller one (2), and so on.
– Continue until you’ve merged all elements.
4. Final Sorted List:
– The merged list is now [1, 2, 3, 4, 5, 6, 7].
Why Merge Sort is Efficient:
Merge Sort’s efficiency comes from its ability to break down a large sorting problem into smaller, more manageable parts. Each sub-list is independently sorted, making the overall sorting process faster and more organized.
Let’s consider an unsorted array of integers and write a simple C++ program to perform Merge Sort.
#include <iostream>
using namespace std;
// Merge function to merge two sorted arrays
void merge(int arr[], int left, int mid, int right) {
int n1 = mid – left + 1;
int n2 = right – mid;
// Create temporary arrays
int L[n1], R[n2];
// Copy data to temporary arrays L[] and R[]
for (int i = 0; i < n1; i++)
L[i] = arr[left + i];
for (int j = 0; j < n2; j++)
R[j] = arr[mid + 1 + j];
// Merge the temporary arrays back into arr[left..right]
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
// Copy the remaining elements of L[], if there are any
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
// Copy the remaining elements of R[], if there are any
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
// Merge Sort function
void mergeSort(int arr[], int left, int right) {
if (left < right) {
// Find the middle point
int mid = left + (right – left) / 2;
// Recursively sort the first and second halves
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
// Merge the sorted halves
merge(arr, left, mid, right);
}
}
// Driver code to test the example
int main() {
int arr[] = {12, 11, 13, 5, 6, 7};
int arrSize = sizeof(arr) / sizeof(arr[0]);
cout << “Unsorted array: “;
for (int i = 0; i < arrSize; i++)
cout << arr[i] << ” “;
// Perform Merge Sort
mergeSort(arr, 0, arrSize – 1);
cout << “\nSorted array: “;
for (int i = 0; i < arrSize; i++)
cout << arr[i] << ” “;
return 0;
}
“`
Explanation:
– The program defines a `merge` function to merge two sorted arrays and a `mergeSort` function to implement the Merge Sort algorithm.
– In the `main` function, an unsorted array is declared, and before and after applying Merge Sort, the array is printed to showcase the sorting process.
Overview of Merge Sort Algorithm
While Merge Sort is already efficient with a time complexity of O(n log n), there are ways to optimize its performance further. One such optimization is the use of insertion sort for small sub-arrays. When the size of the sub-array becomes small enough, switching to insertion sort can reduce the overhead of recursive calls.
Additionally, optimizations like avoiding unnecessary copying during the merging phase can contribute to improved efficiency. Instead of creating new sub-arrays in each recursive call, we can modify the original array in-place by utilizing two auxiliary arrays to represent the left and right halves.
Here’s an optimized version of the `merge` function:
“`cpp
void mergeOptimized(std::vector<int>& arr, int left, int middle, int right) {
int n1 = middle – left + 1;
int n2 = right – middle;
std::vector<int> L(n1), R(n2);
for (int i = 0; i < n1; i++)
L[i] = arr[left + i];
for (int j = 0; j < n2; j++)
R[j] = arr[middle + 1 + j];
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
// Copy the remaining elements if any
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void mergeSortOptimized(std::vector<int>& arr, int left, int right) {
const int INSERTION_THRESHOLD = 10;
if (left < right) {
if (right – left + 1 <= INSERTION_THRESHOLD) {
// Use insertion sort for small sub-arrays
insertionSort(arr, left, right);
} else {
int middle = left + (right – left) / 2;
mergeSortOptimized(arr, left, middle);
mergeSortOptimized(arr, middle + 1, right);
mergeOptimized(arr, left, middle, right);
}
}
}
void insertionSort(std::vector<int>& arr, int left, int right) {
for (int i = left + 1; i <= right; i++) {
int key = arr[i];
int j = i – 1;
while (j >= left && arr[j] > key) {
arr[j + 1] = arr[j];
j–;
}
arr[j + 1] = key;
}
}
“`
In the optimized version:
– The `mergeSortOptimized` function checks if the size of the sub-array is below a certain threshold (in this case, 10 elements) and switches to insertion sort for smaller sub-arrays.
– The `insertionSort` function efficiently handles the sorting of small sub-arrays.
Advantages of Merge Sort
1. Easy to Understand:
Merge sort is a straightforward sorting algorithm to grasp. It divides the unsorted list into smaller parts, sorts them individually, and then combines them in a sorted manner.
2. Consistent Performance:
It guarantees consistent O(n log n) time complexity for the worst, average, and best-case scenarios. This means it performs well across a variety of input cases.
3. Stable Sorting:
Merge sort is a stable sorting algorithm, which means that if two elements have equal keys, their relative order is maintained in the sorted output. This is important in scenarios where maintaining the original order of equal elements matters.
4. Efficient for Linked Lists:
Unlike some other sorting algorithms, merge sort works well with linked lists. It doesn’t require random access to elements, making it suitable for scenarios where accessing elements sequentially is more efficient.
5. Divide and Conquer Strategy:
Merge sort follows a “divide and conquer” strategy, breaking down the sorting problem into smaller, more manageable sub-problems. This simplifies the overall sorting process and makes it easier to implement.
6. No In-Place Sorting:
While some sorting algorithms operate in-place (i.e., they rearrange elements within the array without requiring additional memory), merge sort uses additional space for merging. This can be an advantage in situations where memory usage is not a critical constraint.
7. Predictable Behavior:
Merge sort behaves predictably regardless of the input data. This reliability is beneficial in applications where the algorithm’s performance needs to be consistent under different circumstances.
Conclusion
In conclusion, the merge sort algorithm offers a powerful solution for optimizing sorting processes in various applications. Its efficiency, scalability, and stability make it a favorable choice for handling large datasets. By carefully analyzing its time complexity and implementing best practices, developers can further enhance its performance. Embracing the potential of merge sort allows us to streamline operations, expedite data processing, and ultimately unlock new possibilities in the realm of sorting algorithms.