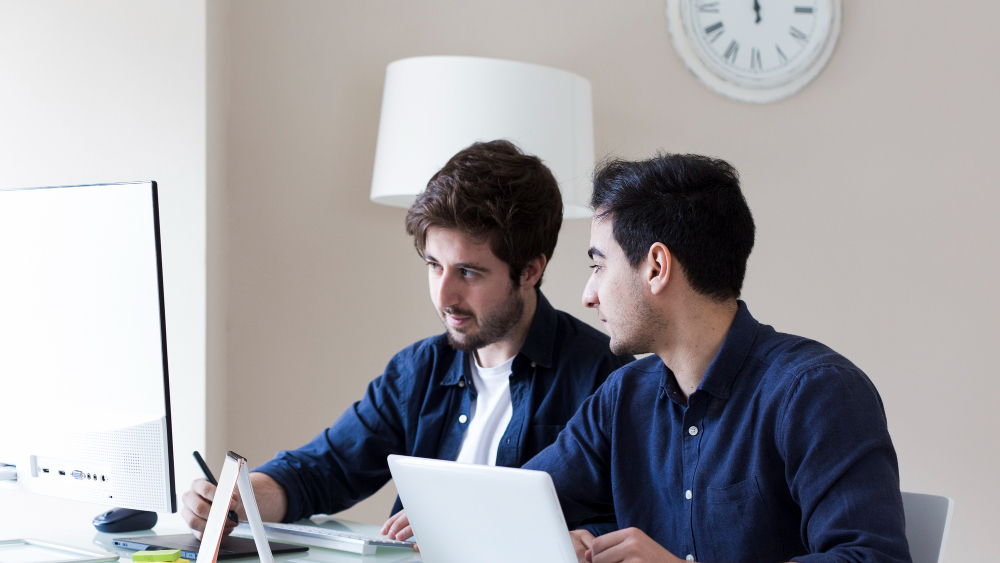
Hey there, future coding rockstars! Welcome back to the T1 Coding Challenge series. Today, we’re tackling Challenge #2, designed to assess your grasp of fundamental programming concepts. Don’t worry if you’re a first-year engineering student – this challenge is all about building a strong foundation.
What’s a Coding Challenge?
Before we dive in, let’s talk about coding challenges in general. These are practical tests that assess your programming skills. Companies often use them during the hiring process to evaluate how you approach problems and translate them into code.
Why are Coding Challenges Important?
Here’s the deal: Resumes can only tell you so much. Coding challenges provide a hands-on way for companies to see your problem-solving skills in action. They assess your ability to think logically, write efficient code, and understand core programming concepts.
So, what is T1 Coding Challenge 2 all about?
This challenge focuses on two key areas: variables and basic operations.
- Variables: Imagine a box that can hold different values. In programming, variables act like those boxes. You give them a name (like “age”) and assign a value (like 25) to them. You can then use these variables in your code to manipulate that data.
- Basic Operations: These are the building blocks of any program – addition (+), subtraction (-), multiplication (*), and division (/).
The Challenge:
The specific prompt for T1 Coding Challenge 2 might vary, but here’s a general example:
Write a program that calculates the total cost of a purchase, including a base price and a sales tax. The user should be able to enter the base price and the tax rate (as a percentage).
Breaking it Down:
- Define Variables:
- We need two variables: one to store the base price (basePrice) and another to hold the tax rate (taxRate). These will be floating-point numbers (numbers with decimals) since prices and percentages usually involve decimals.
- Get User Input:
- We need to ask the user to enter the base price and tax rate. We can use functions like input() (in Python) or prompt() (in Javascript) to capture their input and convert it to floating-point numbers using float().
- Calculate the Tax Amount:
- Use the basic math operation of multiplication to calculate the tax amount.
taxAmount = basePrice * taxRate / 100
- Here, we divide the tax rate by 100 to convert it into a decimal value (e.g., 10% becomes 0.1) before multiplying by the base price.
- Calculate the Total Cost:
- Another simple addition operation! Add the base price and the calculated tax amount to get the final total cost.
totalPrice = basePrice + taxAmount
- Display the Result:
- Finally, use a function like print() (Python) or console.log() (Javascript) to display the calculated total cost to the user.
Example Code (Python):
basePrice = float(input(“Enter the base price: “))
taxRate = float(input(“Enter the tax rate (as a percentage): “))
taxAmount = basePrice * taxRate / 100
totalPrice = basePrice + taxAmount
print(“The total cost, including tax, is:”, totalPrice)
Tips for Success:
- Read the prompt carefully: Make sure you understand what the challenge asks you to do.
- Break it down: Divide the problem into smaller, manageable steps.
- Comment your code: Add comments to explain what each line of code does. This will make it easier for you and others to understand your logic.
- Test your code: Run your code with different inputs to ensure it produces the correct results.
Conclusion:
Mastering the basics is crucial for becoming a proficient programmer. T1 Coding Challenge 2 is a great way to practice working with variables, basic operations, and user input. Even if you encounter a different challenge prompt, the core concepts remain the same.
Don’t be afraid to experiment, try different approaches, and learn from your mistakes. The more you practice, the more comfortable and confident you’ll become with coding fundamentals. And remember, tools like CodexPro can be a valuable asset in your coding journey, helping you assess your skills and prepare for real-world coding challenges. Keep coding, future engineers, and good luck!