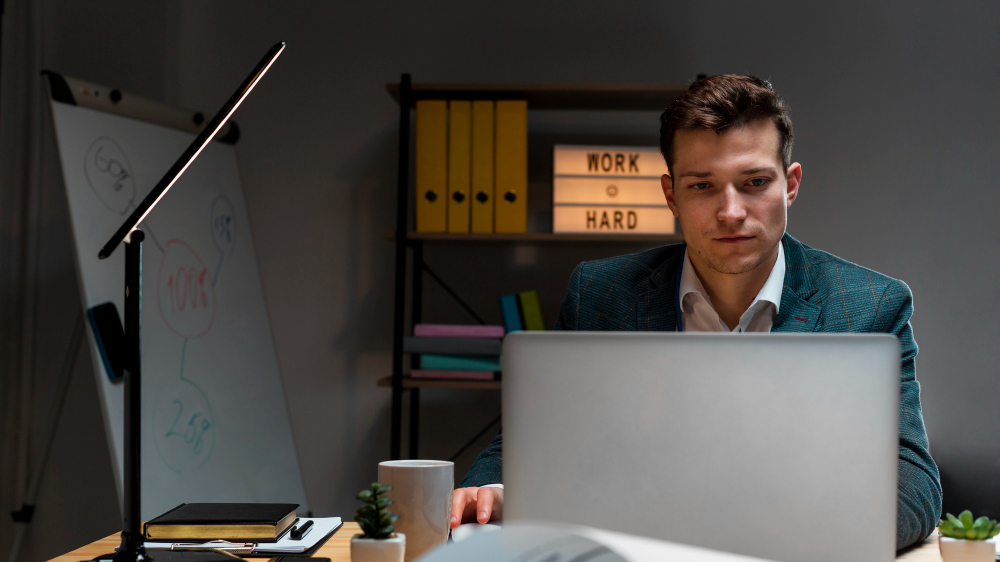
Building a strong foundation in coding is key, and showcasing your skills through well-crafted challenges can make you stand out. This blog dives into the world of simple coding challenges, providing you with the tools to impress recruiters and land that coveted engineering role.
Why Coding Challenges Matter
Think of coding challenges as mini-adventures in the land of programming. They allow you to:
- Practice core concepts: These challenges solidify your understanding of fundamental programming principles like loops, conditional statements, functions, and data structures.
- Develop problem-solving skills: Coding is all about breaking down problems into smaller, solvable steps. Each challenge refines your ability to approach and conquer coding obstacles.
- Showcase your abilities: Adding completed challenges to your portfolio demonstrates your coding proficiency to potential employers. Platforms like CodexPro ([CodexPro.ai]) can even streamline the process for recruiters to assess your skills.
- Prepare for technical interviews: Many companies use coding challenges during the interview stage. By practicing beforehand, you’ll feel more confident and prepared to tackle these assessments.
Let’s Get Coding! Examples of Simple Challenges
Now, let’s dive into some specific examples of simple coding challenges perfect for first-year engineering students. We’ll explore these challenges in Python, a popular and beginner-friendly language:
- Challenge 1: Reversing a Number
This challenge tests your grasp of loops and string manipulation. Here’s the prompt:
Write a program that takes a positive integer as input and prints the reversed version of that number.
Example:
number = int(input(“Enter a positive integer: “))
reversed_number = 0
while number > 0:
remainder = number % 10
reversed_number = (reversed_number * 10) + remainder
number //= 10
print(“Reversed number:”, reversed_number)
- Challenge 2: Checking for Palindromes
This challenge combines the concepts of string manipulation and conditional statements. Here’s the prompt:
Write a program that determines if a given string is a palindrome. A palindrome is a word that reads the same backward as forward (e.g., “racecar”, “level”).
Example:
text = input(“Enter a word or sentence: “)
# Convert to lowercase and remove spaces for case-insensitive check
clean_text = text.lower().replace(” “, “”)
# Check if the reversed version is the same as the original
is_palindrome = clean_text == clean_text[::-1] # Slicing reverses the string
if is_palindrome:
print(text, “is a palindrome”)
else:
print(text, “is not a palindrome”)
- Challenge 3: Calculating Grades
This challenge introduces the concept of conditional statements and user input. Here’s the prompt:
Write a program that takes a student’s score as an input and outputs their corresponding letter grade (A, B, C, D, F) based on a predefined grading scale (e.g., A: 90-100, B: 80-89, etc.).
Example:
score = int(input(“Enter your score: “))
if score >= 90:
grade = “A”
elif score >= 80:
grade = “B”
elif score >= 70:
grade = “C”
elif score >= 60:
grade = “D”
else:
grade = “F”
print(“Your grade is:”, grade)
Tips and Resources for Success
- Start simple and gradually increase the complexity: Don’t overwhelm yourself. Begin with basic challenges and work your way up.
- Practice regularly: Consistency is key! Dedicate some time daily or weekly to hone your coding skills.
- Don’t be afraid to experiment: Coding is about finding solutions. Play around with the code, try different approaches, and learn from your mistakes.
- Seek help when needed: There’s a vast online community of programmers willing to assist. Utilize forums like Stack Overflow (https://stackoverflow.com/) to get help with specific problems.
- Explore online platforms: Platforms like HackerRank, LeetCode, and (of course) CodexPro provide a vast library of coding challenges with varying difficulty levels.
Conclusion: Mastering the Art of Code
By embracing simple coding challenges, you’ll transform yourself from a coding novice to a confident programmer ready to tackle real