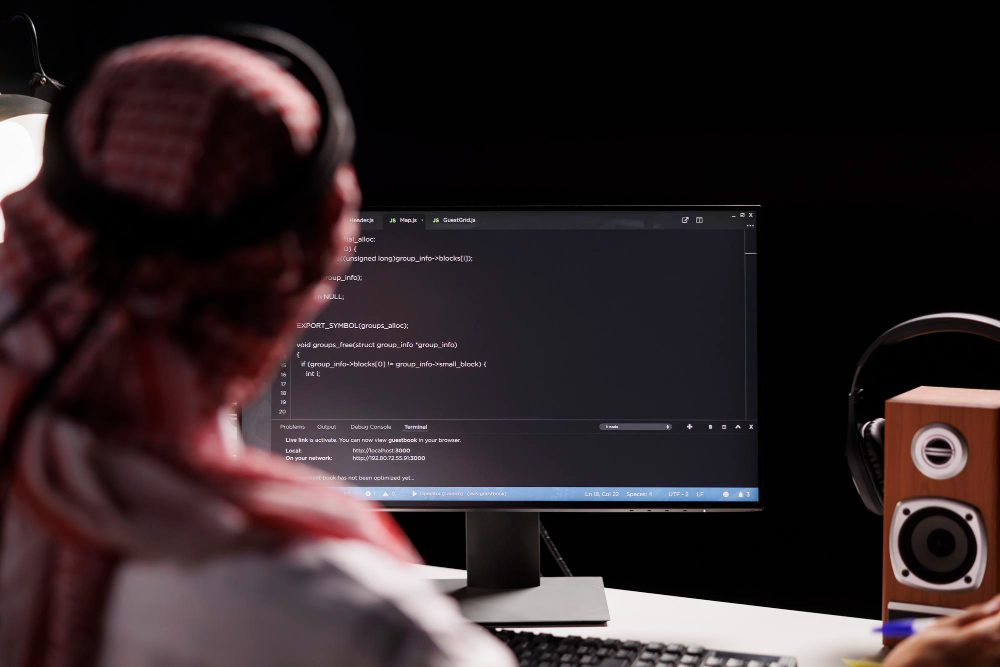
Welcome to the exciting world of Python! This beginner-friendly language is a popular choice for first-time programmers due to its readability and versatility. Whether you’re aiming for a career in software development or simply want to add coding skills to your arsenal, Python is a fantastic place to start.
This blog post serves as your launchpad, packed with essential Python questions designed to solidify your understanding of core concepts. We’ll explore variables, data types, operators, control flow, and more, all with clear explanations and practical examples. So, grab your favorite code editor (like Visual Studio Code or PyCharm) and let’s dive in!
1. Variables and Data Types: Storing Information
Imagine variables as storage boxes for your data. You can assign a name (variable) to a specific value, and Python remembers it for later use. But data comes in different forms, requiring different “boxes.” This is where data types come into play.
Example:
name = “Alice” # String data type (text)
age = 25 # Integer data type (whole numbers)
gpa = 3.8 # Float data type (decimal numbers)
is_enrolled = True # Boolean data type (True or False)
2. Operators: Performing Calculations and Comparisons
Think of operators as tools that manipulate your data. Python offers various operators for performing calculations, comparisons, and more.
Example:
# Arithmetic operators: +, -, *, /, // (integer division), % (modulo)
total_cost = price * quantity
discounted_price = price – (price * discount / 100)
# Comparison operators: == (equal), != (not equal), <, >, <=, >=
is_adult = age >= 18
is_eligible = gpa > 3.5 and is_enrolled
# Logical operators: and, or, not
has_experience = has_python_skills or has_java_skills
3. Control Flow: Making Decisions and Repeating Tasks
Control flow statements allow your code to make decisions (like “if” statements) and repeat actions (like “for” and “while” loops). Mastering these is crucial for writing dynamic programs.
Example:
# If statement:
grade = “A”
if grade == “A”:
print(“Excellent work!”)
# For loop:
for i in range(1, 6): # Loops 5 times (from 1 to 5)
print(f”Iteration {i}”)
# While loop:
guess = 0
while guess != secret_number: # Loops until the guess is correct
guess = int(input(“Guess the number: “))
print(“You guessed it right!”)
4. Functions: Reusable Code Blocks
Imagine writing the same code repeatedly. Functions come to the rescue! They are reusable blocks of code that perform a specific task, making your code cleaner and more organized.
Example:
def greet(name): # Function definition
print(f”Hello, {name}!”)
greet(“Bob”) # Function call
def calculate_area(length, width):
return length * width
area = calculate_area(5, 3)
print(f”The area is {area}”)
5. Lists and Tuples: Collections of Data
Sometimes, you need to store multiple related pieces of information. Lists and tuples come into play as ordered collections. Lists are mutable (changeable), while tuples are immutable (unchangeable).
Example:
# List:
shopping_list = [“apples”, “bread”, “milk”]
shopping_list.append(“eggs”) # Modifying a list
# Tuple:
coordinates = (10, 20) # Tuples cannot be changed
Conclusion:
By tackling these questions and experimenting. The journey to becoming a coding master is a continuous learning process. So, buckle up, have fun with these problems, and get ready to impress recruiters with your sharp programming skills.